If you are still looking for some options, there is a NPM package that might help you, a drop-in replacement for console.log called console-log-json. The nice thing about it is that it will create consistent JSON formatted logs, despite the fact that you can throw at console.log() any number of parameters in any order, including other JSON objects as well as Error objects and plain strings.
It also handles automatically adding some extra helpful attributes such as time stamps and file where the console.log() was called from, etc..
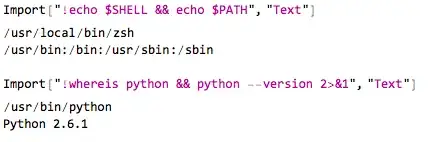
These all get printed to STDOUT so any log shipping and aggregator can pick it up and parse the JSON.