I'm having a problem with TextFields inside a LazyColumn.
My goal is to have a LazyColumn with a bunch of TextFields where I can
- scroll to see the whole content of the LazyColumn even if the keyboard is active
- enter a new line to a TextField and that line is not hidden by the keyboard
- enter Text and the keyboard does not get closed immediately
Thats my code:
LazyColumn(
modifier = Modifier.background(Color.Blue)
) {
items(count = 20, key = { it }) {
var text by remember { mutableStateOf("$it.") }
OutlinedTextField(
value = text,
onValueChange = { text = it },
modifier = Modifier
.fillMaxWidth()
.padding(2.dp)
.background(Color.Gray)
)
}
}
Problem:
When using this code the keyboard will hide all new lines when the TextField is at the bottom of the Column. You can manually scroll down and see the new lines, but thats not an ideal solution. Also, if there are more Textfields underneath it they are hidden and can't be reached by scrolling, so if I'd like to enter text in TextField 17 first and TextField 18 afterwards I have to close the keyboard in between.

Attempt:
I tried to solve this by setting android:windowSoftInputMode="adjustResize"
in the AndroidManifest, but when I click on a TextField at the bottom of the screen the LazyColumn scrolls and the keyboard gets closed.
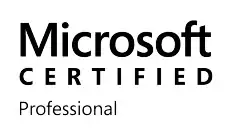
There is a nice explanation of this behaviour here and the suggestion is
Now, workarounds include using a column, instead of a lazy column; creating your own lazy column using a custom lazy Composable, and manually handling the heap size for the Composable so the final field doesn't go out of composition.
Assuming I have a huge number of TextFields and can't use a Column, is there any other way then creating a custom LazyColumn?
This sounds like a really complicated way of solving an issue that I'd expect quite often (or is placing TextFields inside LazyColumns that uncommon?)
Thank you very much for your help! :)