It's really important to make your questions reproducible to get the best answers quickly. In lieu of your data, I created some.
library(gt)
library(tidyverse)
coln = stringi::stri_rand_strings(10, 10, pattern = "[a-z]")
df1 <- map_dfr(1:10,
function(j) {
set.seed(j)
res <- sample(rep(c(TRUE, FALSE), 10), 10) # random select 10
setNames(res, coln) # create a data frame row
})
Right now my data fields all have logical values. If your TRUE
is really a string, you'll need to use "TRUE"
instead of TRUE
.
Since you have multiple columns and any that have true are those you wanted to change, you can simplify it by selecting all columns individually and for each testing for rows that match TRUE
. I used lapply
to create the locations.
First, here's a function I'll use to create a list of column and row locations to test in my table for conditional formatting.
selector <- function(x, stng = TRUE) {
cells_body(columns = !!sym(x),
rows = !!sym(x) == stng)
}
Now I'll use the function tab_style
to create the styles for my conditionally selected cells. You didn't specify whether you wanted to change the color of the text or the cell background. This first example sets the text colors, where coln
is a list of my column names.
df1 %>% gt() %>%
tab_style(style = cell_text(color = "green"), # color text
locations = lapply(coln, selector))
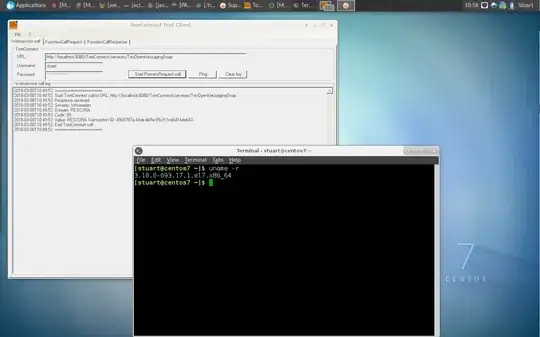
If the true/false values in your table are documented as strings, then in lapply
, you need to add a string to replace the default value of stng
, i.e., lapply(coln, selector, "TRUE")
If you wanted to set the cell background to green, you would change cell_text
to cell_fill
.
df1 %>% gt() %>%
tab_style(style = cell_fill(color = "green"), # color background
locations = lapply(coln, selector))
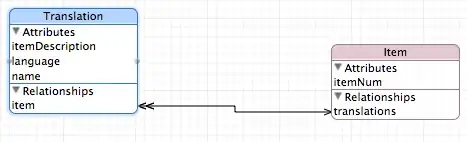
If you don't have the names of your columns separated, there is no need to create another object, just use names()
with your data frame. Here's an example of using names
along with using alpha
or opacity.
df1 %>% gt() %>%
tab_style(style = cell_fill(color = "green", alpha = .2),
locations = lapply(names(df1), selector))
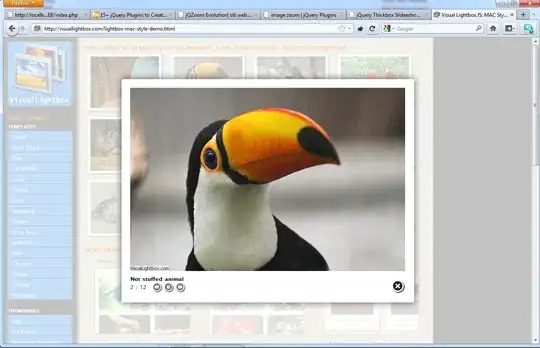