You should really embrace Python libraries to perform certain tasks for which code is already tested and neatly packaged into a library. For your purposes (importing data, array manipulation and operations and plotting) libraries such as Pandas, Numpy and Matplotlib are very common and powerful, there are a bunch of resources on it's capabilities. I really recommend you to check out their websites:
On Airfoil tools you can directly download a .dat
file which you can import directly using pandas. Then using simple parameters declared when calling your function will correctly parse the data and return it in the appropriate data type (float
instead of str
).
Code Sample:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
filename = 'airfoil.dat' #enter your file path here
data = pd.read_table(filename,delim_whitespace=True,skiprows=[0],names=['x','y'],index_col=False)
#Plot
plt.plot(data.x, data.y, 'r',marker='.',markeredgecolor='black', markersize=3)
plt.plot(0.5*data.x+0.3,0.5*data.y) #Scale & translate the datapoints
#Plot settings
plt.axis('equal')
plt.xlim((-0.05, 1.05))
plt.legend(['GOE 383 AIRFOIL','SCALED AIRFOIL'])
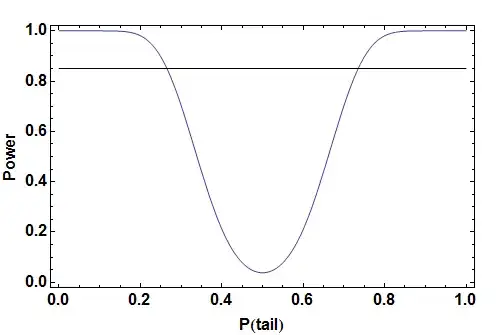