I created a timeline similar to the images you provided using mostly grid
.
In the example I created, there are 3 custom utilities:
main.css:
@tailwind base;
@tailwind components;
@tailwind utilities;
@layer utilities {
.borderGray {
@apply border-y-[1px] border-[lightgray] text-center;
}
.textClass {
@apply m-2 break-words border-2 border-black text-[0.8rem] sm:text-sm md:text-base;
}
.slantedLine {
background: linear-gradient(
to top left,
rgba(0, 0, 0, 0) 0%,
rgba(0, 0, 0, 0) calc(50% - 1px),
lightgray 50%,
rgba(0, 0, 0, 0) calc(50% + 1px),
rgba(0, 0, 0, 0) 100%
);
}
}
- The
borderGray
class creates a gray border on the top and bottom of an element and centers the text to the middle.
- The
textClass
takes care of the font-size
responsiveness. This class can be changed entirely. It just looked convenient here.
- The
slantedLine
class creates a diagonal line crossing the element from the bottom-left corner to the upper-right corner. Here's the answer I used to get it: draw diagonal lines in div background with CSS
Now, to the HTML
.
I created three different variations that can be changed from one to the other depending on the screen size, in case you would like to use more than one variation in your responsive design.
This is what those variations look like:
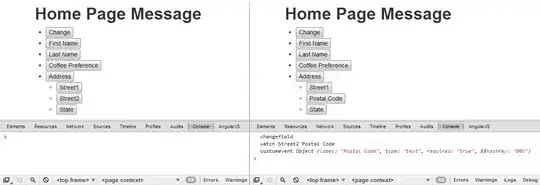
This is what it looks like under the hood:
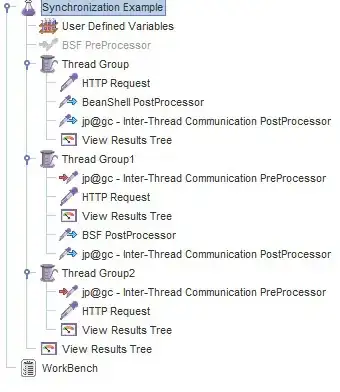
HTML:
<div class="m-12 grid grid-cols-7 grid-rows-4 border-2 border-red-500 bg-slate-100">
<div class="borderGray row-start-4"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine row-start-4"></div>
<div class="slantedLine col-start-2 row-start-3"></div>
<div class="borderGray col-start-3 row-start-3"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-4 row-start-3"></div>
<div class="slantedLine col-start-4 row-start-2"></div>
<div class="borderGray col-start-5 row-start-2"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-6 row-start-2"></div>
<div class="slantedLine col-start-6"></div>
<div class="borderGray"><p class="textClass">Some Text/Link</p></div>
</div>
<div class="m-12 grid grid-cols-11 grid-rows-4 border-2 border-red-500 bg-slate-100">
<div class="borderGray col-span-2 row-start-4"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine row-start-4"></div>
<div class="slantedLine col-start-3 row-start-3"></div>
<div class="borderGray col-span-2 col-start-4 row-start-3"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-6 row-start-2"></div>
<div class="slantedLine col-start-6 row-start-3"></div>
<div class="borderGray col-span-2 col-start-7 row-start-2"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-9"></div>
<div class="slantedLine col-start-9"></div>
<div class="borderGray col-span-2 col-start-10 row-start-1"><p class="textClass">Some Text/Link</p></div>
</div>
<div class="grid-cols-15 m-12 grid grid-rows-4 border-2 border-red-500 bg-slate-100">
<div class="borderGray col-span-3 row-start-4"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-4 row-start-4"></div>
<div class="slantedLine col-start-4 row-start-3"></div>
<div class="borderGray col-span-3 col-start-5 row-start-3"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-8 row-start-2"></div>
<div class="slantedLine col-start-8 row-start-3"></div>
<div class="borderGray col-span-3 col-start-9 row-start-2"><p class="textClass">Some Text/Link</p></div>
<div class="slantedLine col-start-12"></div>
<div class="slantedLine col-start-12"></div>
<div class="borderGray col-span-3 col-start-13 row-start-1"><p class="textClass">Some Text/Link</p></div>
</div>
Tailwind-play
Explanation:
There are three differences between the variations:
- The
col-span-{n}
of each text's container. Docs
- The
grid-cols-{n}
and grid-rows-{n}
. Docs-1 Docs-2
- The
col-start-{n}
and row-start-{n}
of each element inside the grid
. Docs-1 Docs-2
Let's take the second variation to demonstrate. Let's take off the classes to understand how it works:
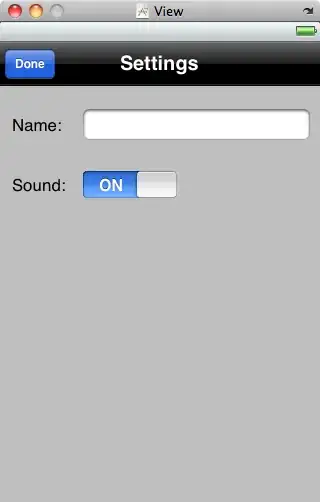
Each odd-numbered element is simply the area for the text. We insert a paragraph in those divs to show the desired text/link:
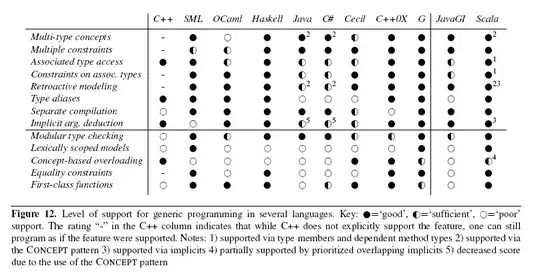
Each even-numbered element is an area for the diagonal class (slantedLine
). The diagonal line goes from the bottom-left corner to the upper-right corner. When we put two elements with this kind of line on each other, we get the effect of a diagonal border on the top and bottom:
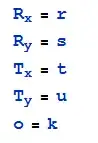
And together:
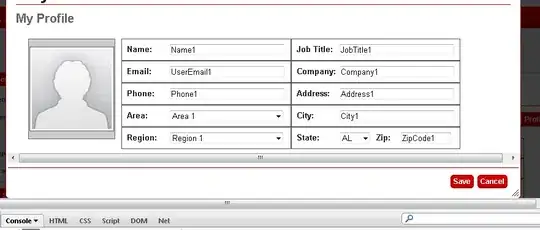
It might not give you the exact design you were looking for, but it can give you an idea of how to progress from here.
Let me know if you have questions.
I hope it helped.