You can put your ViewPager and HorizontalPagerIndicator inside a box with contentAlignment = Alignment.Center/TopCenter/BottomCenter
.
@Composable
private fun AlignmentSample() {
Box(contentAlignment = Alignment.Center) {
HorizontalPager(()
HorizontalPagerIndicator()
}
}
What i mean in the answer in the link you shared is some modifiers are unique to scopes of some Composables. For instance Modifier.align() is available is only inside Box. because of that you either need to place your content inside Box
or you need to set a receiver to your @Composable argument such as
@Composable
private fun BoxScopeSample(
modifier: Modifier = Modifier,
content: @Composable BoxScope.() -> Unit
) {
// You can't call content() here because it has BoxScope receiver
Box(modifier) { // this here is BoxScope
content()
}
}
inside content lambda you can call Modifier.align() which is unique to BoxScope and use it as
BoxScopeSample(modifier = Modifier.size(200.dp).border(2.dp, Color.Cyan)) {
Row(
Modifier
.align(Alignment.TopStart)
.background(Color.Red)
.size(100.dp)
) {
}
Row(
Modifier
.align(Alignment.BottomEnd)
.background(Color.Green)
.size(100.dp)
) {
}
}
Which will look as
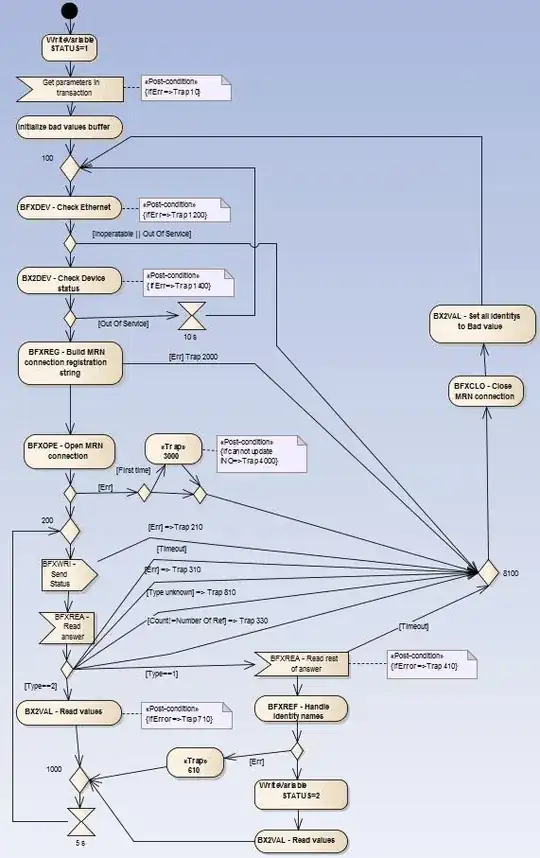
Then create another Composable with no scope such as
@Composable
private fun NonScopeSample(
modifier: Modifier = Modifier,
content: @Composable () -> Unit
) {
Box(modifier) {
content()
}
}
And try calling
NonScopeSample(modifier = Modifier
.size(200.dp)
.border(2.dp, Color.Cyan)) {
Row(
Modifier
.align(Alignment.TopStart)
.background(Color.Red)
.size(100.dp)
) {
}
Row(
Modifier
.align(Alignment.BottomEnd)
.background(Color.Green)
.size(100.dp)
) {
}
}
you will get compile error because Modifier.align is not a generic Modifier as i mentioned above.