Yes, it's possible.
To change the size of an external app window you should use Win32 APIs: SetWindowPos
or MoveWindow
. In fact, your question has already been (partially) answered here.
To access those APIs you can use win32-api. Here's a quick example of resizing a window to 600x600 using the window class name:
const Win32 = require('win32-api/promise');
const User32 = Win32.User32.load(['FindWindowExW','SetWindowPos']);
const SWP_NOMOVE = 0x0002, SWP_NOACTIVATE = 0x0010;
module.exports = {
ResizeWindow: async function ResizeWindow(className)
{
const lpszClass = Buffer.from(className + '\0', 'ucs2')
const hWnd = await User32.FindWindowExW(0, 0, lpszClass, null); // https://learn.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-findwindowexw
User32.SetWindowPos(hWnd, 0, 0, 0, 600, 600, SWP_NOMOVE | SWP_NOACTIVATE); // https://learn.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-setwindowpos
}
};
For MS PPT 2013, the class name is PPTFrameClass
. You can also use the process ID to get the main window handle like this.
And here's a quick demo:
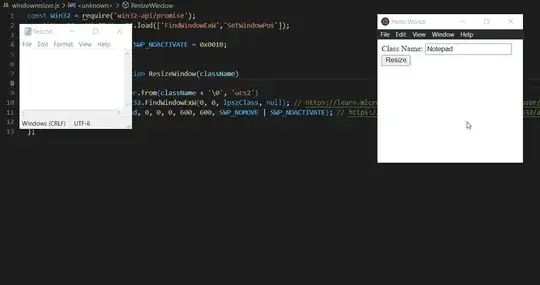
EDIT:
These are the versions that worked for me:
C:\Users\abc>node -v
v18.12.1
"devDependencies": {
"electron": "^19.0.0"
},
"dependencies": {
"ffi-napi": "^4.0.3",
"ref-union-di": "^1.0.1",
"win32-api": "^20.1.0"
}