I have to use large title (navigationBarTitleDisplayMode = .large) and .searchable in View 2.
But in that case, the height of the navigation bar in View 3 is set strangely.
I think, the sum of the height of the navigation bar and the height of the search bar in View 2 is applied to the height of the navigation bar in View 3.
Is there a way to set the height of the navigation bar in view 3 to the height it would have when .inline?
import SwiftUI
struct ContentView: View {
init() {
let appearance = UINavigationBarAppearance()
appearance.configureWithOpaqueBackground()
appearance.backgroundColor = UIColor.red
UINavigationBar.appearance().standardAppearance = appearance
UINavigationBar.appearance().compactAppearance = appearance
UINavigationBar.appearance().scrollEdgeAppearance = appearance
}
var body: some View {
NavigationView {
ScrollView {
VStack {
NavigationLink("View 2") {
SecondView()
}
}
}
.navigationTitle("View 1")
.navigationBarTitleDisplayMode(.large)
}
}
}
struct SecondView: View {
@State var text = ""
var body: some View {
ScrollView {
VStack {
NavigationLink("View 3") {
ThirdView()
}
}
}
.navigationTitle("View 2")
.navigationBarTitleDisplayMode(.large)
.searchable(text: $text)
}
}
struct ThirdView: View {
var body: some View {
Text("View 3")
.navigationTitle("View 3")
.navigationBarTitleDisplayMode(.inline)
}
}
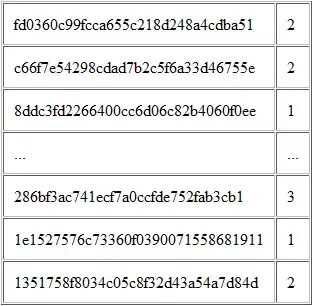