We could add window listener with JavaScript using driver.execute_script
to listen to any clicks, and then call function xpath
as provided in SO answer to generate Xpath of an element. As a gist, below is the window.addEventListener
script which handles any click
event by displaying an alert with the clicked element text (if present) and its Xpath:
window.addEventListener('click', function(event) {alert(event.target.text+'=>'+xpath(event.target));})
And here is the relevant code to launch the browser, execute the script and sleep for 20 seconds to allow interaction on the browser:
def launch_url(url):
driver = webdriver.Chrome('./chromedriver')
driver.get(url)
driver.execute_script('''
function xpath(el) {
if (typeof el == 'string') return document.evaluate(el, document, null, 0, null);
if (!el || el.nodeType != 1) return '';
if (el.id) return '//*[@id="' + el.id + '"';
var sames = [].filter.call(el.parentNode.children, function (x) { return x.tagName == el.tagName });
return xpath(el.parentNode) + '/' + el.tagName.toLowerCase() + (sames.length > 1 ? '['+([].indexOf.call(sames, el)+1)+']' : '')
}
window.addEventListener('click', function(event) {alert(event.target.id+'=>'+xpath(event.target));})
''')
time.sleep(20)
As a test, launched the SO main questions page with launch_url("https://stackoverflow.com/questions")
and clicked on the "Ask Question" button:
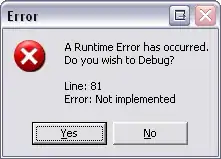