You can use ImageMagick in the Terminal/shell:
magick INPUT.JPG -compress none OUTPUT.PPM
If you omit -compress none
you'll get binary (i.e. P6) PPM output.
If using old v6 ImageMagick, that becomes:
convert INPUT.JPG -compress none OUTPUT.PPM
All the options, switches, operators and settings for ImageMagick are documented here.
If you want to convert PPM to JPEG, or to PNG, you can just use:
magick INPUT.PPM OUTPUT.JPG
or
magick INPUT.PPM OUTPUT.PNG
You can also programmatically create a random PPM file like this:
#!/bin/bash
W=5; H=4
echo "P3\n${W} ${H}\n255" > image.ppm
for ((i=0;i<$((W*H*3));i++)) ; do
echo $((RANDOM%255))
done >> image.ppm
Then enlarge for easy viewing and make into a PNG:
magick image.ppm -scale 200x result.png
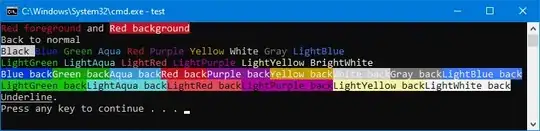
Or, the same thing again, nut maybe slightly more elegantly and without creating an intermediate file:
#!/bin/bash
W=5; H=4
{
printf "P3\n${W} ${H}\n255\n"
for ((i=0;i<$((W*H*3));i++)) ; do
echo $((RANDOM%255))
done
} | magick ppm:- -scale 200x result.png
If you prefer to use the lighter weight, but far less capable NetPBM tools, it would be:
jpegtopnm -plain INPUT.JPG > OUTPUT.PPM