I have created a desktop WinForm application using .NET 6 framework and write the following code.
I hope this code will help you in some way to fix your problem.
Note: you have to use XmlAttribute
Namespace
properly to fix your missing attribute issue.
However, the starting p: will stay remain missing.
EidBusinessSearch class:
[XmlRoot(ElementName = "EIDVBusinessSearch", Namespace = "example.com")]
public class EIDVBusinessSearch
{
[XmlElement(ElementName = "PermissiblePurpose", Namespace = "")]
public PermissiblePurpose? PermissiblePurpose { get; set; }
[XmlAttribute(AttributeName = "p", Namespace = "http://www.w3.org/2000/xmlns/")]
public string? P { get; set; }
[XmlAttribute(AttributeName = "xsi", Namespace = "http://www.w3.org/2000/xmlns/")]
public string? Xsi { get; set; }
[XmlAttribute(AttributeName = "schemaLocation", Namespace = "abc.com")]
public string? SchemaLocation { get; set; }
[XmlText]
public string? Text { get; set; }
}
PermissiblePurpose
[XmlRoot(ElementName = "PermissiblePurpose", Namespace = "")]
public class PermissiblePurpose
{
[XmlElement(ElementName = "GLB", Namespace = "")]
public string? GLB { get; set; }
[XmlElement(ElementName = "DPPA", Namespace = "")]
public string? DPPA { get; set; }
[XmlElement(ElementName = "VOTER", Namespace = "")]
public string? VOTER { get; set; }
}
Method to Convert XML to Object:
private void ConvertXMLtoObject()
{
try
{
string xml = "<p:EIDVBusinessSearch xmlns:p=\"example.com\" xmlns:xsi=\"abc.com\" xsi:schemaLocation=\"cde.com\">" +
"\r\n <PermissiblePurpose>" +
"\r\n <GLB>{{GLB}}</GLB>" +
"\r\n <DPPA>{{DPPA}}</DPPA>" +
"\r\n <VOTER>{{VOTER}}</VOTER>" +
"\r\n </PermissiblePurpose>" +
"\r\n</p:EIDVBusinessSearch>";
XmlSerializer serializer = new(typeof(EIDVBusinessSearch));
EIDVBusinessSearch obj = new();
using StringReader reader = new(xml);
obj = (EIDVBusinessSearch)serializer.Deserialize(reader);
}
catch (Exception ex)
{
Console.WriteLine("Error: " + ex.Message);
Application.Exit();
}
}
Convert Object to XML:
private void ConvertObjectToXML()
{
try
{
EIDVBusinessSearch obj = new()
{
P = "example.com",
PermissiblePurpose = new()
};
obj.PermissiblePurpose.GLB = "GLB";
obj.PermissiblePurpose.DPPA = "DPPA";
obj.PermissiblePurpose.VOTER = "VOTER";
XmlSerializer serializer = new(obj.GetType());
using StringWriter writer = new();
serializer.Serialize(writer, obj);
string xml = writer.ToString();
label1.Text = xml;
}
catch (Exception ex)
{
Console.WriteLine("Error: " + ex.Message);
Application.Exit();
}
}
Output:
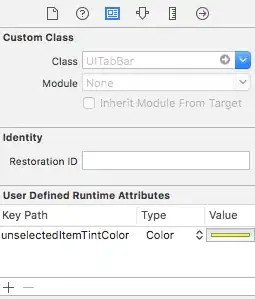