So you said your app is in python, and you are trying to publish it to the web, you don't have to convert your code to Javascript, you can create a WebApp/WebApi application, it is like making a website, but all the website will do is the conversion, everything else remains in python, I will suggest you go the web API route, that will be simpler, Python Has A FastAPI (https://pypi.org/project/fastapi/) module you can use, you can watch this tutorial on it, the first 8 min of the tutorial is more than enough if you still need help, let me know (https://www.youtube.com/watch?v=VSQZl43jFzk)
But I still got your code to work on my side, at this point the project is not a python project anymore, I won't recommend you continue on this path but here is the code if you feel like seeing how it will work.
This is the code
<!DOCTYPE html>
<html>
<head >
<title>Title of the document</title>
</head>
<body>
The content of the document......
</body>
<script>
// Print the welcome message
console.log('||| Welcome to our app which shows the ounce prices and optimum prices of homeland gold coins.||| \n||| Use the numbers for type of gold coins below ||| \n||| Cumhuriyet(1),Tam(2),Yarım(3),Çeyrek(4),Finish the calc and show sum(5) ||| ');
console.log('||| This is a calc which calculates the entries you have entered by order.||| \n||| To quit enter the type of gold 5 and amount of gold is any number. |||');
// Collect data from web
async function getData() {
const cors_bypass = "https://cors-anywhere.herokuapp.com/"; //Had to add this line because I was getting a CORS error
const url = cors_bypass + 'https://yorum.altin.in/tum/ons';
const headers = { 'User_Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/100.0.4896.88 Safari/537.36','method' : "GET", 'Accept': '*/*', 'mode':'no-cors'};
console.log(headers);
const page = await fetch(url, { headers });
const content = new DOMParser().parseFromString(await page.text(), 'text/html');
const x = content.getElementById('ofiy').innerText.trim();
const y = content.getElementById('dfiy').innerText.trim();
const a = parseFloat(x.substring(1, 9));
const c = parseFloat(y.substring(0, 6));
const ws = [0.212666471635951 * a * c, 0.206772168098369 * a * c, 0.103386084049185 * a * c, 0.0516930420245924 * a * c];
const calcx = [];
return { "calcx" : calcx, "ws" : ws, "c":c};
}
// Define the function that calculates the price of the gold coins
// Define the main loop
async function main() {
let q = parseInt(prompt('enter representive number of your coin: '));
let wx = parseInt(prompt('enter amount of gold which related to q value: '));
return { "q" : q , "wx" : wx};
}
function pr_gold( q , wx , calcx, ws, c) {
console.log(q);
if (q === 1) {
calcx.push(ws[0] * parseInt(wx));}
else if (q === 2) {
calcx.push(ws[1] * parseInt(wx));}
else if (q === 3) {
calcx.push(ws[2] * parseInt(wx));}
else if (q === 4) {
calcx.push(ws[3] * parseInt(wx));}
else if (q === 5) {
console.log(`||| Calculation is completed. USD/TRY parity is ${c} TRY and ounce price of gold is ${a} USD |||`);
console.log(`||| The total value of your Turkish gold coins are ${Math.ceil(calcx.reduce((a, b) => a + b, 0))} TRY |||`);}
else {
console.log("Invalid input. Please enter a number between 1 and 5.");}
}
var getdata_results = {};
getData().then((result) =>{
getdata_results = result;
//console.log(result);
main().then((results) => {
main_results = results;
//console.log(results);
pr_gold( main_results["q"], main_results["wx"], getdata_results["calcx"], getdata_results["ws"], getdata_results["c"]);
});
} );
</script>
</html>
When I run the code, I inputted 2 and 2 for q and wx and I got the following :
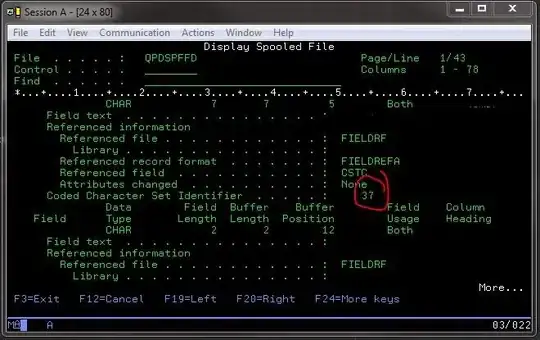
So the above code works for the input q=2 and wx=2 other inputs might give you an error, but I am still debugging it. let me know if you are interested in the refined code that works for all scenarios. The following inputs q=5 and wx=2 will give you an error, just saying before you think my code does not work, I fixed the code to work on the bare minimum.
Yeah, So I Got A CORS Error, if you run my code and also get the CORS error, visit this site https://cors-anywhere.herokuapp.com/corsdemo there will be a button that says "request temporary access to demo server" click it then rerun the code.
Due to the fact that you made your functions async, I had to use the ".then()" notation to get this to work, I would suggest you learn about async stuff, anyone reading this should please link to some async materials. It's good to use async but first make sure you need it, I feel like I can write this code in a more beginner-friendly manner without async.
Looking at the code, it looks like whoever wrote it hasn't yet grasped the workings of variable scopes, the user @jabaa https://stackoverflow.com/users/16540390/jabaa posted a link to materials on JS async stuff, I will just post it here for convenience
Does this answer your question?https://stackoverflow.com/questions/500431/what-is-the-scope-of-variables-in-javascript
Yeah, So any further questions, post below, looking at the code, I feel like whoever wrote this code needs 1 or 2 weeks more of tutorials into the Javascript Basics.
I did not explain much of the changes I did, because well, it will be like I am writing documentation, and that's not my favorite thing to do, so post in the comment below as to whether I should explain my changes, then I will do it ( i will only do that if the request comes from @lex ).