The issue with accessing the Script_Instance
from your custom class is independent of accessing the Rhino libraries from a custom class.
To access the Script_Instance
methods, you can pass the object into your method and add the static
tag.
For accessing rhino libraries there is no special inheritance.
The example blow demonstrates both implementations:
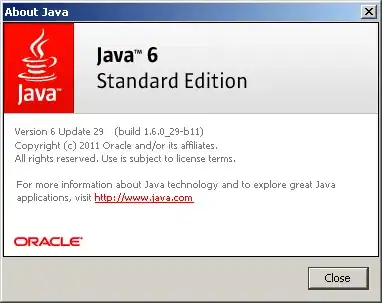
private void RunScript(List<Point3d> PointsList, ref object NurbSrf, ref object SrfArea)
{
Print("Example:");
//Access script object from custom class.
MyClass.TestFoo(this);
//Access Rhino doc in custom class.
string docName = NurbsHelpers.ActiveDocName();
string objectCount = NurbsHelpers.ActiveDocObjectCount();
Print("doc '" + docName + "' has [" + objectCount + "] objects in it");
//Use Nurbs Methods in custom class.
NurbsSurface surface = NurbsHelpers.MakeNurbs(PointsList);
double area = NurbsHelpers.NurbsArea(surface);
//Assign script component outputs.
NurbSrf = surface;
SrfArea = area;
//Bake surface into active doc
NurbsHelpers.bakeNurbSrf(surface);
}
// <Custom additional code>
public class MyClass
{
public static void TestFoo(Script_Instance script)
{
script.Print("it works!");
}
}
public class NurbsHelpers
{
public static NurbsSurface MakeNurbs(List<Point3d> Points){
NurbsSurface surface = NurbsSurface.CreateFromCorners(
Points[0],
Points[1],
Points[2],
Points[3]
);
return (surface);
}
public static double NurbsArea(NurbsSurface surface){
Brep surfaceBrep = surface.ToBrep();
return surfaceBrep.GetArea();
}
public static string ActiveDocName(){
return Rhino.RhinoDoc.ActiveDoc.Name;
}
public static string ActiveDocObjectCount(){
return Rhino.RhinoDoc.ActiveDoc.Objects.Count.ToString();
}
public static void bakeNurbSrf(NurbsSurface nurbSrf){
Rhino.RhinoDoc doc = Rhino.RhinoDoc.ActiveDoc;
doc.Objects.AddSurface(nurbSrf);
}
}