Based on the following using directives:
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
it seems that you may be attempting to display a DataTable
on a WebForm
.
Prerequisite
If developing on Win 10, ensure that IIS is enabled/installed (Control Panel => View by: Small icons => Programs and Features => Turn Windows features on or off => check "Internet Information Services" => expand "Internet Information Services" => expand "World Wide Web Services" => expand "Application Development Features" => check "ASP.NET 4.8" => click "OK")
Try the following:
VS 2022:
Create a new ASP.NET Web Application (.NET Framework)
Open Visual Studio 2022
Click Create a new project

For filter, select
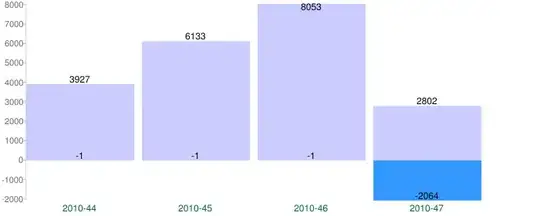
Select ASP.NET Web Application (.NET Framework)
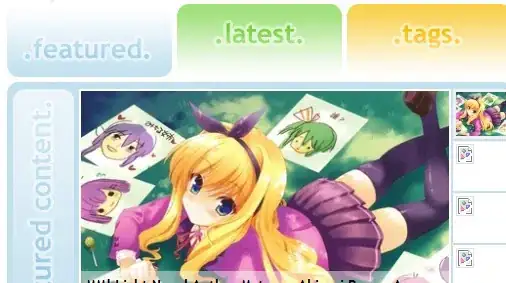
Click Next
Enter desired project name (ex: WebAppGridViewTest)
Select desired framework (ex: .NET Framework 4.8)
Click Create
On the "Create a new ASP.NET Web Application" form, select Empty.
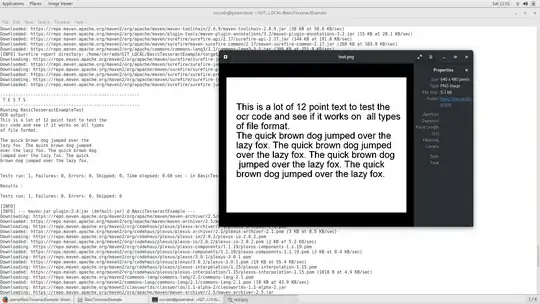
If you're using "http" instead of "https", under "Advance", uncheck "Configure for HTTPS".
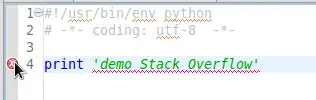
Click Create
Add Web Form to project
- In VS menu, click Project
- Select Add New Item...
- On left side, expand "Web". Select Web Form (name: default.aspx)
- Click Add
Open Solution Explorer
- In VS menu, click View
- Select Solution Explorer
default.aspx:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="default.aspx.cs" Inherits="WebAppGridViewTest._default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="LabelMsg" runat="server" Text="" style="position:absolute;left:50px; top:60px"></asp:Label>
</div>
<div style="position:absolute;left:50px; top:100px">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" DataKeyNames="ID" GridLines="Both">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID" ItemStyle-HorizontalAlign="Center" Visible="True" />
<asp:BoundField DataField="FirstName" HeaderText="First Name" ItemStyle-HorizontalAlign="Center" />
<asp:BoundField DataField="LastName" HeaderText="Last Name" ItemStyle-HorizontalAlign="Center" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
In Solution Explorer, expand "default.aspx" and select "default.aspx.cs".
default.aspx.cs
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
namespace WebAppGridViewTest
{
public partial class _default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
DataTable dt = new DataTable();
dt.Columns.Add(new DataColumn("ID", typeof(int)));
dt.Columns.Add(new DataColumn("FirstName", typeof(string)));
dt.Columns.Add(new DataColumn("LastName", typeof(string)));
//add test data
AddRow(dt, 1, "John", "Doe");
AddRow(dt, 2, "Tom", "Smith");
AddRow(dt, 3, "Susan", "Archer");
GridView1.DataSource= dt;
GridView1.DataBind();
}
private void AddRow(DataTable dt, int id, string firstName, string lastName)
{
DataRow row = dt.NewRow();
row["ID"] = id;
row["FirstName"] = firstName;
row["LastName"] = lastName;
//add
dt.Rows.Add(row);
}
}
}
Result:
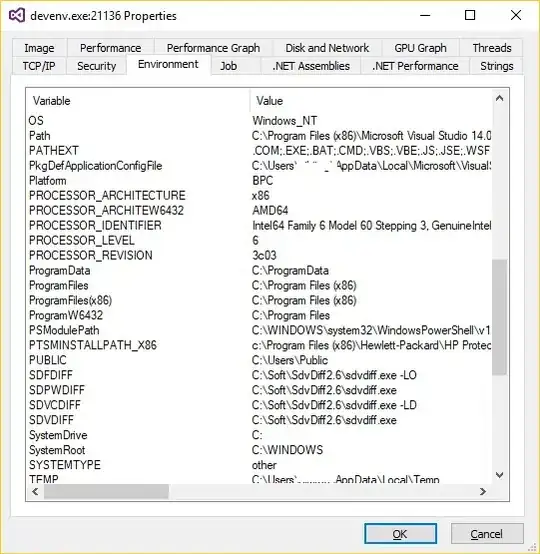
Resources