Use createMany to achieve this on relationShips:
https://laravel.com/docs/9.x/eloquent-relationships#the-create-method
If you want to do this on the model directly, you can just create the function:
ExampleModel:
public static function createMany($records){
foreach($records as $record) {
$this->create($record);
}
}
If you look at the create() function in the model:
Illuminate\Database\Eloquent\Builder;
/**
* Save a new model and return the instance.
*
* @param array $attributes
* @return \Illuminate\Database\Eloquent\Model|$this
*/
public function create(array $attributes = [])
{
return tap($this->newModelInstance($attributes), function ($instance) {
$instance->save();
});
}
it uses the save() function that dispatches the event "creating"
: https://laravel.com/docs/9.x/eloquent#events
Save() will call $this->performInsert($query)
and
performInsert does $this->fireModelEvent('creating')
as well as $this->updateTimestamps()
As @Techno mentioned: https://laravel.com/docs/9.x/eloquent#upserts:~:text=When%20issuing%20a,a%20mass%20update
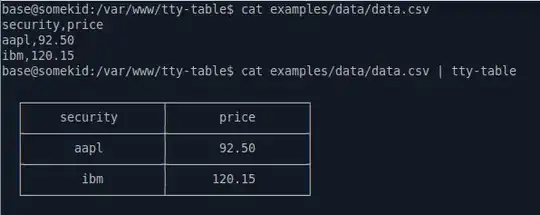