Your question is how to bind DataGridView to specific properties . You mention that you have a collection of Data
but don't say whether it's observable (for example BindingList<Data>
). You end your post with what's the easiest way to do this? While that's a matter of opinion, one way that I personally find very easy is to allow AutoGenerateColumns
and do column formatting on a bindable source in the OnLoad
override of the form.
Example
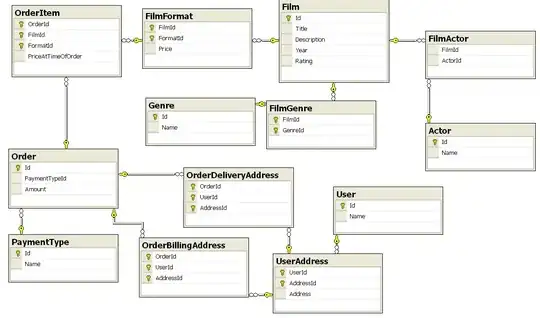
public partial class MainForm : Form
{
public MainForm() => InitializeComponent();
internal BindingList<Data> Rows { get; } = new BindingList<Data>();
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
dataGridView.AllowUserToAddRows = false;
dataGridView.DataSource = Rows;
dataGridView.CellEndEdit += (sender, e) => dataGridView.Refresh();
#region F O R M A T C O L U M N S
Rows.Add(new Data()); // <= Auto-generate columns
dataGridView.Columns["A"].Width = 50;
dataGridView.Columns["A"].DefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleCenter;
dataGridView.Columns["A"].HeaderCell.Style.Alignment = DataGridViewContentAlignment.MiddleCenter;
dataGridView.Columns["C"].AutoSizeMode = DataGridViewAutoSizeColumnMode.Fill;
dataGridView.Columns["C"].HeaderCell.Style.Alignment = DataGridViewContentAlignment.MiddleCenter;
Rows.Clear();
#endregion F O R M A T C O L U M N S
// Add a few items
Rows.Add(new Data { A = 1, B = 1000L });
Rows.Add(new Data { A = 2, B = 2000L });
Rows.Add(new Data { A = 3, B = 3000L });
}
}
The Data
class defines whether the column is shown and editable using visibility and attributes.
class Data
{
// A visible, editable cell.
public int A { get; set; }
// Non-visible because property is declared as internal.
internal long B { get; set; }
// Visible, read-only cell that dynamically responds
// when cell 'A" is edited due to Refresh()
public string C => $"A={A} B={B}";
// Non-visible because of attribute.
[Browsable(false)]
public string? D { get; set; } = "Not visible";
}
Another alternative, provided the column exists in the first place, is that it can be shown-hidden. For example: dataGridView.Columns["C"].Visible = false
.