You shouldn't change a list while you're iterating though it. Trying to create fancy list comprehensions is unlikely to help you especially if you're learning. If you're still really keen, look up the build in function map() instead on how to apply a function to an iterable.
Here is an example that adds random rectangles to a list on mouse-clicks and then draws them all:
import random
import pygame
WIDTH = 640
HEIGHT = 480
FPS = 30
pygame.init()
window = pygame.display.set_mode((WIDTH, HEIGHT))
shapes = []
clock = pygame.time.Clock()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONUP:
x = random.randint(0, WIDTH - 1) # randint includes endpoints
y = random.randint(0, HEIGHT - 1)
shape = pygame.Rect(x, y, random.randint(20, 100), random.randint(20, 100))
shapes.append(shape)
# draw surface - fill background
window.fill(pygame.color.Color("grey"))
## draw shapes
for shape in shapes:
pygame.draw.rect(window, "firebrick", shape)
# show surface
pygame.display.update()
# limit frames
clock.tick(FPS)
pygame.quit()
If you run this code and click a few times, note that the scroll wheel can generate lots of clicks, you'll see something like this:
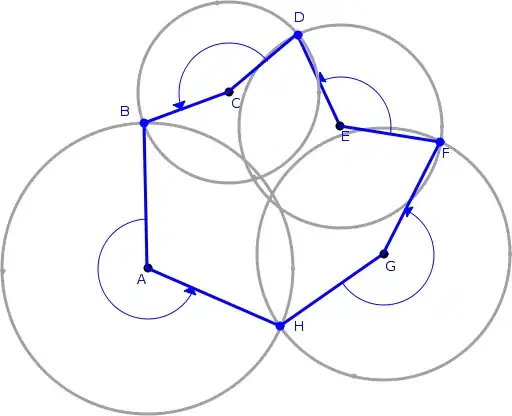
If you want to do things more complicated, for example, make each rectangle a different color you can create sprites to control your shapes and store information and sprite groups to easily control them. Here's an example.