In Windows OS (and only for 32 bits Python versions), you can use 'unicurses' module. You can download it from pypi.org (https://pypi.org/project/UniCurses/1.2/) and install it as a .exe file.
when you have already installed it, it will appear an 'import module error', all about it is explained on this post here in SOF just in case: UniCurses pdcurses.dll Error
Basically you have to download 'pdcurses.dll' (here is a link: https://www.opendll.com/index.php?file-download=pdcurses.dll&arch=32bit&version=3.4.0.0) and move it to a specific directory. It's detalled on the post above.
Here is an example code of how this module works to use it with Arrow Keys:
from unicurses import *
WIDTH = 30
HEIGHT = 10
startx = 0
starty = 0
choices = ["Choice 1", "Choice 2", "Choice 3", "Choice 4", "Exit"]
n_choices = len(choices)
highlight = 1
choice = 0
c = 0
def print_menu(menu_win, highlight):
x = 2
y = 2
box(menu_win, 0, 0)
for i in range(0, n_choices):
if (highlight == i + 1):
wattron(menu_win, A_REVERSE)
mvwaddstr(menu_win, y, x, choices[i])
wattroff(menu_win, A_REVERSE)
else:
mvwaddstr(menu_win, y, x, choices[i])
y += 1
wrefresh(menu_win)
stdscr = initscr()
clear()
noecho()
cbreak()
curs_set(0)
startx = int((80 - WIDTH) / 2)
starty = int((24 - HEIGHT) / 2)
menu_win = newwin(HEIGHT, WIDTH, starty, startx)
keypad(menu_win, True)
mvaddstr(0, 0, "Use arrow keys to go up and down, press ENTER to select a choice")
refresh()
print_menu(menu_win, highlight)
while True:
c = wgetch(menu_win)
if c == KEY_UP:
if highlight == 1:
highlight == n_choices
else:
highlight -= 1
elif c == KEY_DOWN:
if highlight == n_choices:
highlight = 1
else:
highlight += 1
elif c == 10: # ENTER is pressed
choice = highlight
mvaddstr(23, 0, str.format("You chose choice {0} with choice string {1}", choice, choices[choice-1]))
clrtoeol()
refresh()
else:
mvaddstr(22, 0, str.format("Character pressed is = {0}", c))
clrtoeol()
refresh()
print_menu(menu_win, highlight)
if choice == 5:
break
refresh()
endwin()
And here is an image of the result on the command line interface (CMD):
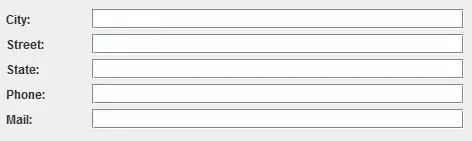