Since you can use .NET in x++, it is pretty similar. However, you have to give up some syntactic sugar and deal with some oddities on how x++ deals with .NET types and objects. This is why usually, such code is not written directly in x++, but in a C# client that is in turned called from x++.
In case you really want to do this in x++, here is some code for Dynamics 365 Finance and Operations where I tried to recreate the question's code as faithfully as possible in x++. I added some comments to explain some of the differences. If earlier versions (e.g. Dynamics AX 2012) are used, you may need to add some InteropPermission.
Additional information can be found at X++ and C# Comparison and .NET Interop from X++.
using System.Net.Http;
using System.Net.Http.Headers;
internal final class SOSendingAPOSTCommand
{
public static void main(Args _args)
{
// client is a x++ keyword
var httpClient = new HttpClient();
// x++ does not support object initializers, so attributes of new objects need to be set separately
var request = new HttpRequestMessage();
// static methods and fields are called with double colon in x++
request.Method = HttpMethod::Post;
// if using System; is used, the exception type of the info method is not decidable anymore
request.RequestUri = new System.Uri("https://httpbin.org/post");
var stringContent = new StringContent("{\"id\":\"2\",\"name\":\"Karam\"}");
// x++ does not like multiple chained calls with .NET objects, so stringContent.Headers.ContentType cannot be used
var headers = stringContent.Headers;
headers.ContentType = new MediaTypeHeaderValue("application/json");
request.Content = stringContent;
// there is no await or async in x++
var response = httpClient.SendAsync(request).Result;
response.EnsureSuccessStatusCode();
var body = response.Content.ReadAsStringAsync().Result;
info(body);
}
}
When I execute this runnable class on my local VHD environment on version 10.0.29, I get the following text as a result. Note that I'm using https://httpbin.org/post as service to test the POST request because I don't have access to the local http://localhost:8080/users of the question.
{
"args": {},
"data": "{\"id\":\"2\",\"name\":\"Karam\"}",
"files": {},
"form": {},
"headers": {
"Content-Length": "25",
"Content-Type": "application/json",
"Host": "httpbin.org",
"X-Amzn-Trace-Id": "Root=1-63d51491-66f3cd9964eda82b5e496448"
},
"json": {
"id": "2",
"name": "Karam"
},
"origin": "212.117.82.182",
"url": "https://httpbin.org/post"
}
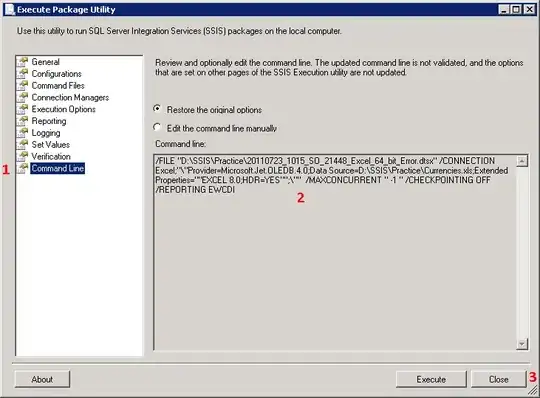