This should be fairly simple:
fig, ax = plt.subplots(1, 2) # or (2, 1) depending on how you want them aligned
ax[0].plot(...)
ax[1].plot(...)
For the dual y-axis, follow this: https://matplotlib.org/stable/gallery/subplots_axes_and_figures/secondary_axis.html
EDIT:
Here is an example, almost directly copied from the above documentation:
import numpy as np
import matplotlib.pyplot as plt
month = np.linspace(1, 12, 12)
DC_high_temp = np.array([43, 47, 56, 67, 76, 85, 89, 87, 81, 69, 59, 48]) # °F
DC_low_temp = np.array([25, 27, 35, 44, 54, 63, 68, 68, 59, 46, 37, 30]) # °F
SEATTLE_high_temp = np.array([46, 50, 54, 58, 64, 69, 73, 74, 69, 59, 50, 45]) # °F
SEATTLE_low_temp = np.array([36, 37, 39, 42, 48, 52, 56, 56, 52, 46, 40, 36]) # °F
def degF_to_degC(T):
return (T - 32) * (5 / 9)
def degC_to_degF(T):
return (T * (9 / 5)) + 32
fig, ax = plt.subplots(2,1)
plt.tight_layout(pad = 2.5)
ax[0].plot(month, DC_high_temp, label = "High Temperature")
ax[0].plot(month, DC_low_temp, label = "Low Temperature")
ax[0].set_ylabel("Temperature (°F)")
ax[0].set_title("DC Average Monthly Temperature")
ax[0].legend()
ax[1].plot(month, DC_high_temp, label = "High Temperature")
ax[1].plot(month, DC_low_temp, label = "Low Temperature")
ax[1].set_ylabel("Temperature (°F)")
ax[1].set_title("Seattle Average Monthly Temperature")
ax[1].set_xlabel("Month")
sec_y_axis_0 = ax[0].secondary_yaxis('right', functions = (degF_to_degC, degC_to_degF))
sec_y_axis_0.set_ylabel("Temperature (°C)")
sec_y_axis_1 = ax[1].secondary_yaxis('right', functions = (degF_to_degC, degC_to_degF))
sec_y_axis_1.set_ylabel("Temperature (°C)")
plt.show()
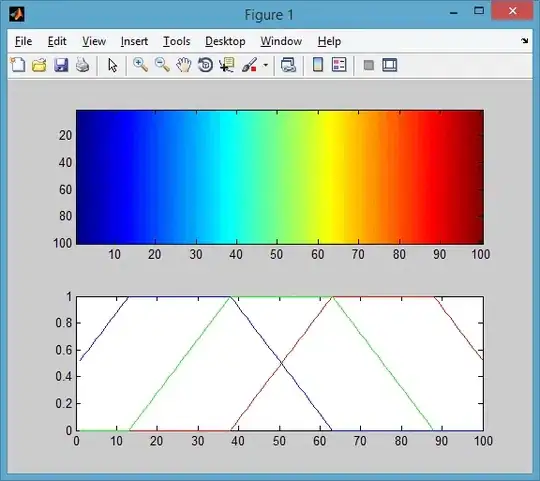