You can use CompositedTransformTarget
and CompositedTransformFollower
as mentioned on comment section by pskink.
class AppPT extends StatelessWidget {
const AppPT({super.key});
@override
Widget build(BuildContext context) {
final LayerLink _layerLink = LayerLink();
return Scaffold(
body: Column(
children: [
Stack(
children: [
Align(
child: CompositedTransformTarget(
link: _layerLink,
child: Container(
color: Colors.red,
width: 100,
height: 60,
alignment: Alignment.center,
child: Text("btn"),
),
),
),
CompositedTransformFollower(
link: _layerLink,
targetAnchor: Alignment.centerRight,
followerAnchor: Alignment.centerLeft,
child: Container(
color: Colors.cyanAccent,
width: 12,
height: 12,
alignment: Alignment.center,
child: Text("*"),
),
),
],
)
],
),
);
}
}
There are few tricks I can think of,
You can use Stack widget with Position.
including another widget on right by applying opacity(invisible) on current snippet.
using transform will be handle if you know the width of the widget.
Transform.translate(
offset: Offset(20 / 2, 0), //20 is the * box size
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
color: Colors.red,
width: 100,
height: 60,
alignment: Alignment.center,
child: Text("btn"),
),
Container(
color: Colors.green,
width: 20,
height: 20,
child: Center(child: Text("*")),
),
],
),
),
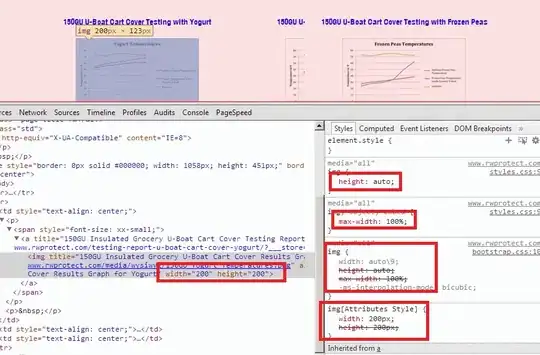