I don't think you will be able to achieve what you want with Excel, because, as you said, the workbook you're trying to access the information from is not open. The easiest way is probably Power Query.
Since you did not post a sample data set, I made one up.
Table 1 (contained in a file I called Book2.xlsx):
+---------+
| MyData1 |
+---------+
| 1000 |
| 1005 |
| 1010 |
| 1015 |
| 1020 |
| 1025 |
| 1030 |
| 1035 |
| 1040 |
| 1045 |
| 1050 |
| 1055 |
| 1060 |
| 1065 |
+---------+
Table 2 (contained in a file I called Book1.xlsx):
+---+
| 1 |
| 2 |
| 3 |
| 4 |
| 5 |
+---+
The goal is to pull the value of "4" into a second column in Book2.xlsx.
For this, I navigated to the "Data" tab in Book1.xlsx and pulled the table into Power Query as a connection only. I called the query "Book1". (In Excel, go to Data > Get Data > From File
, then navigate to the file you want to access.)
I then pulled in the data from Book2.xlsx. When you do this with data that has a header and you mark it as such, Excel automatically converts your range to a table object. Here it's called "Table1", and I left it at that for the query name.
As a last step, I added a custom column as follows in the Table1 query:
= Book1[Column1]{3}
The {3}
is the zero-based position of the value that you want from the other query.
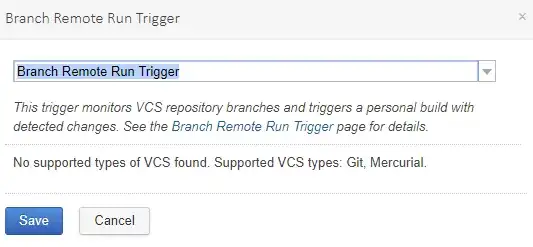
The result is a column that automatically fills each row with the value you specified from the other file, and it does it without the issues you were experiencing due to the other file not being open.
This is the M code for the Book1 query:
let
Source = Excel.Workbook(File.Contents("PATHTOFILE\Book1.xlsx"), null, true),
Sheet1_Sheet = Source{[Item="Sheet1",Kind="Sheet"]}[Data],
#"Changed Type" = Table.TransformColumnTypes(Sheet1_Sheet,{{"Column1", Int64.Type}})
in
#"Changed Type"
Here is the M code for the Table1 query:
let
Source = Excel.CurrentWorkbook(){[Name="Table1"]}[Content],
#"Changed Type" = Table.TransformColumnTypes(Source,{{"MyData1", Int64.Type}}),
#"Added Custom" = Table.AddColumn(#"Changed Type", "FromBook1", each Book1[Column1]{3})
in
#"Added Custom"
A caveat: the path to the first file (the one you want to extract the information from) is hard-coded into the M code, which can cause issues if the file is later moved. There are work-arounds for this, but that goes beyond of the scope of your question.
The result:
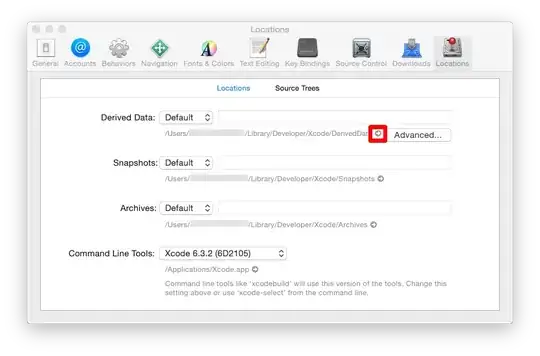