If using another package is OK, {shinyTree} would be an option.
It can use fontAwesome icons or a set of {shinyTree} builtin icons.
You can also use HTML for item labels, thus offering to source your own images.
library(shiny)
library(shinyTree)
ui <- fluidPage(
shinyTree::shinyTree('myShinyTree',
checkbox = TRUE,
themeIcons = FALSE,
theme = 'proton'
)
)
server <- function(input, output, session) {
df <- data.frame(continent = c(rep("Letteria", 2), rep("Numberalia", 1)),
country = c("<img src = 'flag_A.png' width = '50px' /> A-Land",
"<img src = 'flag_B.png' width = '50px' /> B-Country",
"<img src = 'flag_1.png' width = '50px' /> Uni Kingdom"
)
)
output$myShinyTree <- renderTree({
tree_list <- dfToTree(df) ## dfToTree converts df into a hierarch. list
attr(tree_list, 'stopened') <- TRUE
## set the 'stopened' attribute on each node to uncollapse the whole tree
tree_list <- tree_list |>
Map(f = \(.) {attr(., 'stopened') <- TRUE; .})
})
}
shinyApp(ui, server)
Remember to put static content (here: the image files) in a subfolder www
of your app's folder, so that Shiny will find it. Do not include the www
in your path though. Example: reference file www/flag_A.png
as <img src = 'file.png'>
.
Output with custom images:
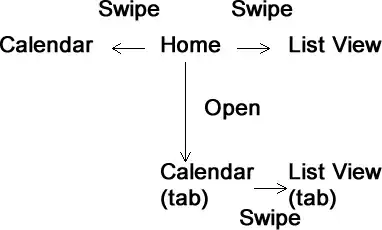
find a repository of country flags here
With lots of symbols it might be convenient to add a class to your images, e.g. <img class='flag' ...>
so that you can apply size, transparency etc. to all flags via CSS.