If you're working with temporary tables and you're on SQL Server 2012+, an option is to use EXECUTE with the RESULT SETS option.
Given your supplied query, I wrapped it with an Execute and specified the column names and types.
EXECUTE(N'
SELECT 1 AS col1, NULL AS col2, NULL AS col3, ''A'' AS col4 INTO #TEMP_1
SELECT NULL AS col1, 2 AS col2, NULL AS col3, ''B'' AS col4 INTO #TEMP_2
SELECT NULL AS col1, NULL AS col2, 3 AS col3, ''John'' AS col4 INTO #TEMP_3
--Creating multiple temp table to increase preforming
--create a final result set
;WITH CTE AS (
SELECT * FROM #TEMP_1
UNION
SELECT * FROM #TEMP_2
UNION
SELECT * FROM #TEMP_3
)
--data I eventually need
SELECT col1,col2,col3
FROM CTE
WHERE 1=1 AND col4=''John''
') WITH RESULT SETS
(
(Column1 int, Column2 int, Column3 int)
);
The OLE DB Source component was able to interpret that metadata just fine and out comes my data.
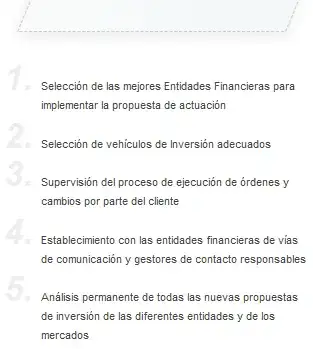
This solution works because it's is dynamic SQL. I am creating a big string and passing it to the EXEC call and specifying the RESULT SET which allows SSIS to properly infer the metadata. If, as I suspect, you need to fool around with dynamic filters and such, then you can do some fancy string building early on and reduce the EXEC call.
-- Do whatever needs to be done to properly define your queries
-- add filtering, etc
-- Before you EXEC, I find printing the resulting SQL handy so I can manually run it through a parser looking for mistakes
DECLARE @DynamicSQL nvarchar(max) = N'
SELECT 1 AS col1, NULL AS col2, NULL AS col3, ''A'' AS col4 INTO #TEMP_1
SELECT NULL AS col1, 2 AS col2, NULL AS col3, ''B'' AS col4 INTO #TEMP_2
SELECT NULL AS col1, NULL AS col2, 3 AS col3, ''John'' AS col4 INTO #TEMP_3
--Creating multiple temp table to increase preforming
--create a final result set
;WITH CTE AS (
SELECT * FROM #TEMP_1
UNION
SELECT * FROM #TEMP_2
UNION
SELECT * FROM #TEMP_3
)
--data I eventually need
SELECT col1,col2,col3
FROM CTE
WHERE 1=1 AND col4=''John''
';
EXECUTE(@DynamicSQL) WITH RESULT SETS
(
(Column1 int, Column2 int, Column3 int)
);
To embed a quote within the existing single quoted mess of statements, you need to double them - two single quotes, not a double