You can use a for loop as u/DanY posted; however, it's harder to store and retrieve plots in a universal way with that structure. Running the loop code makes it difficult to retrieve any one particular plot - you would only see the last plot in the output window and have to go "back" to see the others. I would suggest using a list structure instead to allow you to retrieve any one of the individual plots in subsequent functions.
For this, you can use lapply()
rather than for(...) { ... }
.
Here's an example which uses dplyr
and tidyr
:
library(ggplot2)
library(dplyr)
library(tidyr)
unique_species <- unique(iris$Species)
myPlots <- lapply(unique_species, function(x) {
ggplot(
data = iris %>% dplyr::filter(Species == x),
mapping = aes(x=Sepal.Length, y=Sepal.Width)
) +
geom_point() +
labs(title=paste("Plot of ", x))
})
You then have the plots stored within myPlots
. You can access each plot via myPlots[1]
, myPlots[2]
or myPlots[3]
... or you can plot them all together via patchwork
or another similar package. Here's one way using cowplot
:
cowplot::plot_grid(plotlist = myPlots, nrow=1)
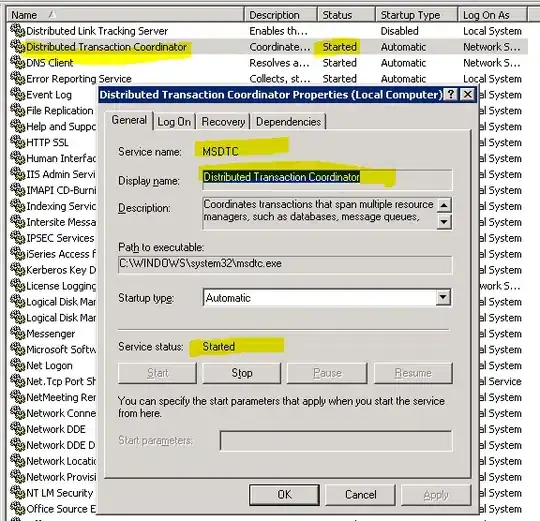