Here's some code to combine arbitrary Geometry objects in GoJS:
function combineGeometries() {
const geo = new go.Geometry();
for (let i = 0; i < arguments.length; i++) {
const g = arguments[i];
if (g instanceof go.Geometry) {
convertToPathGeometry(g).figures.each(f => geo.add(f));
}
}
return geo;
}
function convertToPathGeometry(geo) {
if (geo.type === go.Geometry.Line) {
return new go.Geometry()
.add(new go.PathFigure(geo.startX, geo.startY, false)
.add(new go.PathSegment(go.PathSegment.Line, geo.endX, geo.endY)));
} else if (geo.type === go.Geometry.Rectangle) {
return new go.Geometry()
.add(new go.PathFigure(geo.startX, geo.startY)
.add(new go.PathSegment(go.PathSegment.Line, geo.endX, geo.startY))
.add(new go.PathSegment(go.PathSegment.Line, geo.endX, geo.endY))
.add(new go.PathSegment(go.PathSegment.Line, geo.startX, geo.endY).close()));
} else if (geo.type === go.Geometry.Ellipse) {
const radx = Math.abs(geo.endX - geo.startX)/2;
const rady = Math.abs(geo.endY - geo.startY)/2;
const minx = Math.min(geo.startX, geo.endX);
const maxx = Math.max(geo.startX, geo.endX);
const midy = (geo.startY + geo.endY)/2;
return new go.Geometry()
.add(new go.PathFigure(minx, midy)
.add(new go.PathSegment(go.PathSegment.SvgArc, maxx, midy, radx, rady, 0, true, true))
.add(new go.PathSegment(go.PathSegment.SvgArc, minx, midy, radx, rady, 0, true, true).close()));
} else {
return geo;
}
}
// Example:
const $ = go.GraphObject.make;
const G1 = $(go.Geometry, go.Geometry.Rectangle, { endX: 80, endY: 60 });
const G2 = $(go.Geometry, go.Geometry.Ellipse, { startX: 40, startY: 40, endX: 140, endY: 90 });
const G3 = $(go.Geometry, go.Geometry.Line, { startX: 100, startY: 30, endX: 80, endY: 10 });
const G4 = go.Geometry.parse("M 0 100 L 100 100 100 30 20 30 20 50 70 50 70 80 40 80 40 0 0 0z", true).offset(30, 70);
const G = combineGeometries(G1, G2, G3, G4);
The G4 geometry was taken from https://gojs.net/latest/intro/geometry.html.
When G is used as the Shape.geometry of a Shape, one gets something like:
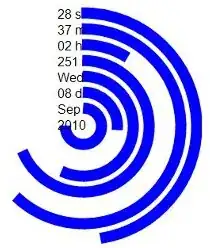
The node template in this case was:
myDiagram.nodeTemplate =
$(go.Node, "Vertical",
$(go.Shape,
{ fill: "white", geometry: G },
new go.Binding("fill", "color")),
$(go.TextBlock,
new go.Binding("text"))
);