Not sure why you need the mouse coordinate, following code demo the way to get it by PySimpleGUI.
import PySimpleGUI as sg
sg.theme("DarkAmber")
layout = [[sg.Text("Mouse Coord:"), sg.Text(size=20, key='Coordinate')]]
window = sg.Window("Mouse Coord", layout, finalize=True)
window.bind('<Motion>', 'Motion')
while True:
event, values = window.read()
if event == sg.WIN_CLOSED:
break
elif event == 'Motion':
e = window.user_bind_event
window['Coordinate'].update(f'({e.x}, {e.y})')
window.close()
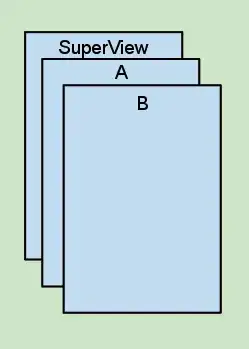
You can get the screen coordinate by e.x_root
and e.y_root
, like
window['Coordinate'].update(f'({e.x_root}, {e.y_root})')
The '<Motion>'
in tkinter only work when mouse on the tkinter window, following code demo the way using pynput
library to capture the motion event, then pass it to PySimpleGUI.
from pynput.mouse import Listener
import PySimpleGUI as sg
def on_move(x, y):
window.write_event_value('Motion', (x, y))
sg.theme("DarkAmber")
layout = [[sg.Text("Mouse Coord:"), sg.Text(size=20, key='Coordinate')]]
window = sg.Window("Mouse Coord", layout, finalize=True, enable_close_attempted_event=True)
listener = Listener(on_move=on_move)
listener.start()
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSE_ATTEMPTED_EVENT:
listener.stop()
break
elif event == 'Motion':
x, y = values[event]
window['Coordinate'].update(f'({x}, {y})')
window.close()