Global variables are frowned upon in programming but this is one area where you can use one to your advantage:
(i) declare the variable at the start of the worksheet module, e.g.
Public prevTarget As String
(ii) initialise it, either manually in the Immediate Window, or programatically in a Workbook_Open()
procedure, e.g.
Sheet1.prevTarget="A1"
(iii) update your Worksheet_Change() procedure
Private Sub Worksheet_Change(ByVal Target As Range)
If Target.Count <> 1 Then Exit Sub
Range(prevTarget).Interior.ColorIndex = xlNone
Target.Interior.ColorIndex = 6
prevTarget = Target.Address
End Sub
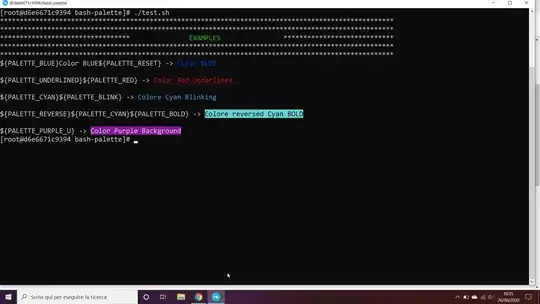
Edited to add history-inclusive code
(i) Declare 2 public variables at the start of the worksheet module, e.g.
Public prevTarget As String, logCount As Long
(ii) Declare a 3rd public variable at the start of an inserted module, e.g.
Public history() As String
(iii) Update the Worksheet_Change() procedure as follows
Private Sub Worksheet_Change(ByVal Target As Range)
If Target.Count <> 1 Then Exit Sub
Application.EnableEvents = False
Application.ScreenUpdating = False
logCount = logCount + 1
ReDim Preserve history(1 To 2, 1 To logCount)
Dim newVal As String
newVal = Target.Formula
Application.Undo
history(1, logCount) = Target.Address
history(2, logCount) = Target.Formula
Target.Formula = newVal
Target.Offset(1, 0).Select 'replicate the effect of cursor movement on Enter (prevented by call to Undo method)
Application.EnableEvents = True
Range(prevTarget).Interior.ColorIndex = xlColorIndexNone
Target.Interior.ColorIndex = 6
prevTarget = Target.Address
Application.ScreenUpdating = True
End Sub
and, whenever you want to view this history, you can execute use this
Sub historyLog()
ActiveCell.Resize(UBound(history, 2), 2).Value2 = Application.Transpose(history)
End Sub
If you make a lot of changes in quick succession then you will notice that data entry is now 'sticky', but that is inevitable because of the call to the Application.Undo()
method, which is required to maintain the change history.
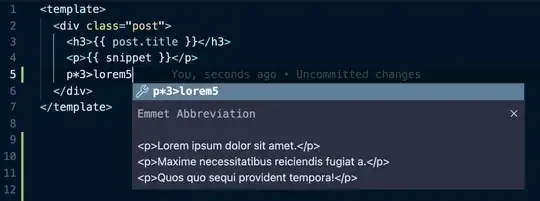