Thanks to qkhanhpro
for the comments.
To have a static variable during the lifetime of the instance, you can store the value in a database or in a file.
And also you can use the environment variable to determine the value of the static variable.
For example, in .NET, you can use the
Environment.GetEnvironmentVariable
method to retrieve the value of the environment variable.
using System;
String envVar = Environment.GetEnvironmentVariable("MY_VAR");
Sample code for Garbage collection
References taken from the MSDoc.
private const long maxGarbage = 1000;
static void Main()
{
Program myGCCol = new Program();
Console.WriteLine("The highest generation is {0}", GC.MaxGeneration);
myGCCol.MakeSomeGarbage();
Console.WriteLine("Generation: {0}", GC.GetGeneration(myGCCol));
Console.WriteLine("Total Memory: {0}", GC.GetTotalMemory(false));
GC.Collect(0);
Console.WriteLine("Generation: {0}", GC.GetGeneration(myGCCol));
Console.WriteLine("Total Memory: {0}", GC.GetTotalMemory(false));
GC.Collect(2);
Console.WriteLine("Generation: {0}", GC.GetGeneration(myGCCol));
Console.WriteLine("Total Memory: {0}", GC.GetTotalMemory(false));
Console.Read();
}
void MakeSomeGarbage()
{
Version vt;
for (int i = 0; i < maxGarbage; i++)
{
vt = new Version();
}
}
Garbage collection
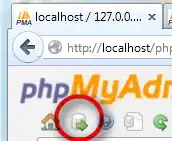
Sample code to check Out of Memory exception
.
try
{
try
{
string s = "This";
s = s.Insert(2, "is ");
throw new OutOfMemoryException();
}
catch (ArgumentException)
{
Console.WriteLine("ArgumentException in String.Insert");
}
}
catch (OutOfMemoryException e)
{
Console.WriteLine("Terminating application unexpectedly...");
Console.ReadLine();
Environment.FailFast(String.Format("Out of Memory: {0}",
e.Message));
}
OOM Exception

For further information refer to on GlobalMemoryStatusEx function and OOM exception.