I think that the count in the Layout inspector is not accurate.
In your code the recomposition occurs only for the Text
inside the Button
.
You can check it visually using this function:
fun randomColor() = Color(
Random.nextInt(256),
Random.nextInt(256),
Random.nextInt(256),
alpha = 255
)
Apply the same function to the background
color of the Button
and text color:
Button(
onClick = {number = Random.nextInt(0,99)},
colors = ButtonDefaults.buttonColors(backgroundColor = getRandomColor())
){
Text("number :$number", color = getRandomColor())
}
You can check that every time you click the Button the Text is recomposed (it changes the text color) due to the number
state that is updated. At the same time the Button is not recomposed.
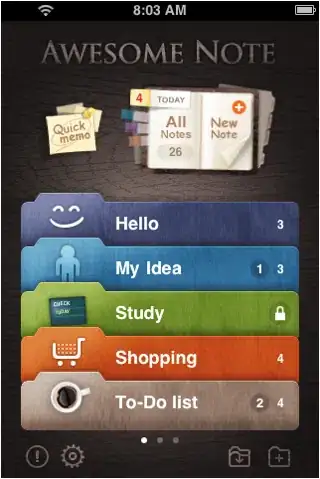
You can also use a Log to check the same result in the Logcat using
class Ref(var value: Int)
// Note the inline function below which ensures that this function is essentially
// copied at the call site to ensure that its logging only recompositions from the
// original call site.
@Composable
inline fun LogCompositions(tag: String, msg: String) {
if (BuildConfig.DEBUG) {
val ref = remember { Ref(0) }
SideEffect { ref.value++ }
Log.d(tag, "Compositions: $msg ${ref.value}")
}
}
and then in your code:
LogCompositions("Myapp", "Button")
Button(
onClick = {number = Random.nextInt(0,99)},
colors = ButtonDefaults.buttonColors(backgroundColor = getRandomColor()),
){
LogCompositions("Myapp", "Text")
Text("number :$number", color = getRandomColor())
}
In the log when the Button
is clicked only the Text
is recomposed:
D Compositions: Text 1
D Compositions: Text 2
D Compositions: Text 3
For more info about the recomposition you can check this great answer by @Thracian and this post about the LogComposition.