Here is what I have tried:
I have used your threshold T
to draw a circle, where the center is base on the nearest point between the points in polygon_1
ad all points in polygon_2
. Then find the intersections, and find the convex hull for the intersection zone. Then find the union of the three polygons, codes :
import numpy as np
import matplotlib.pyplot as plt
from shapely.ops import unary_union
from shapely.geometry import Polygon
from scipy.spatial import ConvexHull
polygon_1 = np.array([[1, 6, 7.8, 7.8, 1, 1], [4, 4, 6, 11, 11, 4]]).T
polygon_2 = np.array([[6, 14, 14, 11, 8.2, 9, 6, 6], [0.5, 0.5, 12, 12, 10, 4.2, 3.5, 0.5]]).T
poly_1 = Polygon(polygon_1)
poly_2 = Polygon(polygon_2)
# define threshold T
T = 2.5
#Initialize a variable to create a circle
theta = np.linspace(0, 2*np.pi, 100)
intersection_points = []
coord_circles = np.zeros((0, 2))
for poly in polygon_1:
# Calculate the distance between the points in polygon_1 ad all points in polygon_2
norm = np.sqrt((poly[0] - polygon_2[:, 0])**2 + (poly[1] - polygon_2[:, 1])**2)
# If the distance is smaaller than the threshold
if np.min(norm) < T:
# Find the nearest point in polygon_2
point_near =polygon_2[ np.argmin(norm)]
x = T*np.cos(theta) + point_near[0]
y = T*np.sin(theta) + point_near[1]
# Crear a polygon for the circle and find intersection points with polygon_1 and polygon_2
poly_circle = Polygon(np.array([x, y]).T)
intersec_1 = poly_1.boundary.intersection(poly_circle.boundary)
intersection_points.append(intersec_1.geoms[0].coords._coords[0])
intersection_points.append(intersec_1.geoms[1].coords._coords[0])
intersec_2 = poly_2.boundary.intersection(poly_circle.boundary)
intersection_points.append(intersec_2.geoms[0].coords._coords[0])
intersection_points.append(intersec_2.geoms[1].coords._coords[0])
intersection_points = np.array(intersection_points)
# Find the Convex hulls
hull = ConvexHull(intersection_points)
# Creat a polygon for the convex hull of the intersections
poly_3 = Polygon(np.c_[intersection_points[hull.vertices,0], intersection_points[hull.vertices,1]])
# Find the union of the these three polygon
a = unary_union([poly_1, poly_2, poly_3])
result_x, result_y = a.exterior.coords.xy
result_x = result_x.tolist()
result_y = result_y.tolist()
plt.subplot(121)
plt.plot(polygon_1[:, 0], polygon_1[:, 1], 'b')
plt.plot(polygon_2[:, 0], polygon_2[:, 1], 'r')
plt.axis('equal')
plt.title("Before merging")
plt.subplot(122)
plt.plot(result_x, result_y, 'k-o')
plt.axis('equal')
plt.title("After merging")
The you get figure :
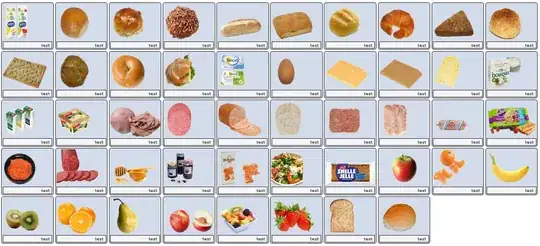