It depends on what you are aiming for.
Here's an experiment that uses various expressions, you can pick one that suits your requirements.
Don't forget the add tbody
to the table selector, since you want to exclude the header row.
Test HTML
<table>
<tbody>
<tr>
<td></td>
<td></td>
</tr>
<tr>
<td><span></span></td>
<td></td>
</tr>
<tr>
<td>some-text</td>
<td></td>
</tr>
<tr>
<td>some-text</td>
<td>another-text</td>
</tr>
</tbody>
</table>
Test code
cy.get('table tbody tr')
.each((tr, index) => {
console.group('row', index, tr.html().replaceAll('\n', ''))
const expressions = [
"tr.html()",
"tr[0].innerHTML",
"tr.find('td').toArray().map(td => td.innerHTML).join('')",
"tr.text()",
"tr.text().trim().replaceAll('\\n', '')",
]
const results = expressions.reduce((acc,e) => {
acc[e] = eval(e)
return acc
}, {})
console.table(results)
console.groupEnd()
})
Output
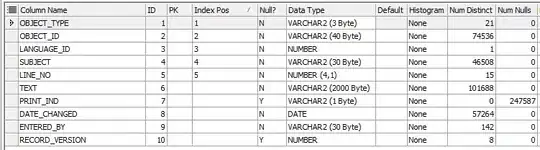