It can be done by adding callbacks to implementation of press and long press by writing your own PointerInputScope function.
Explained in detail how it works here, you can check out if you are interested in details.
suspend fun PointerInputScope.detectPressGestures(
onLongPress: ((Offset) -> Unit)? = null,
onPressStart: ((Offset) -> Unit)? = null,
onPressEnd: (() -> Unit)? = null,
onLongPressEnd: (() -> Unit)? = null,
) = coroutineScope {
awaitEachGesture {
val down = awaitFirstDown()
down.consume()
val downTime = System.currentTimeMillis()
onPressStart?.invoke(down.position)
val longPressTimeout = onLongPress?.let {
viewConfiguration.longPressTimeoutMillis
} ?: (Long.MAX_VALUE / 2)
var longPressInvoked = false
do {
val event: PointerEvent = awaitPointerEvent()
val currentTime = System.currentTimeMillis()
if (!longPressInvoked && currentTime - downTime >= longPressTimeout) {
onLongPress?.invoke(event.changes.first().position)
longPressInvoked = true
}
event.changes
.forEach { pointerInputChange: PointerInputChange ->
pointerInputChange.consume()
}
} while (event.changes.any { it.pressed })
if (longPressInvoked) {
onLongPressEnd?.invoke()
} else {
onPressEnd?.invoke()
}
}
}
Call it inside Modifier.pointerInput(){}
as
Modifier.pointerInput(Unit) {
detectPressGestures(
onPressStart = {
},
onLongPress = {
},
onLongPressEnd = {
},
onPressEnd = {
}
)
}
Full implementation and demo to show color changes on events.
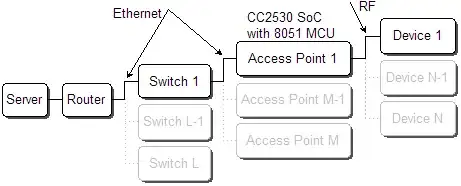
@Preview
@Composable
private fun PressCallbacks() {
var gestureColor by remember { mutableStateOf(Color.LightGray) }
val context = LocalContext.current
Box(modifier = Modifier
.size(200.dp)
.background(gestureColor)
.pointerInput(Unit) {
detectPressGestures(
onPressStart = {
gestureColor = Color.Yellow
Toast
.makeText(
context,
"Press Start",
Toast.LENGTH_SHORT
)
.show()
},
onLongPress = {
gestureColor = Color.Blue
Toast
.makeText(
context,
"Long Press!",
Toast.LENGTH_SHORT
)
.show()
},
onLongPressEnd = {
gestureColor = Color.Black
Toast
.makeText(
context,
"Long Press ENDS",
Toast.LENGTH_SHORT
)
.show()
},
onPressEnd = {
Toast
.makeText(
context,
"Press ENDS",
Toast.LENGTH_SHORT
)
.show()
gestureColor = Color.Cyan
}
)
}
)
}