I have a simple Python script with 3 loops. The loops itself are working without any issue, but I noticed that just the last loop is printing the output in the correct way. The iterations before are showing a line break in the middle of the string, and I don't understand where it is coming from.
# Open the file containing the Keywords
with open('keyword.txt', 'r') as keyword_file:
keywords = keyword_file.readlines()
# Open the file containing the Numbers
with open('number.txt', 'r') as number_file:
numbers = number_file.readlines()
# Loop over each keyword + number + iteration
# outer loop - keywords
for keyword in keywords:
# nested loop - numbers
for number in numbers:
# nested loop - to iterate from 1 to 10
for j in range(1,11):
# print combined string
text = "Keyword: " + str(keyword) + " Number: " + str(number) + " Iteration: " + str(j)
print (text)
# Open a new file to write the combined string
with open('target.txt', 'a') as target_file:
# Write each string to the file on a new line
target_file.write(text + '\n')
The loaded text files are quite basic:
keyword.txt
Word_A
Word_B
Word_C
number.txt
1111
2222
3333
The output in the terminal and also in the target.txt looks like this.
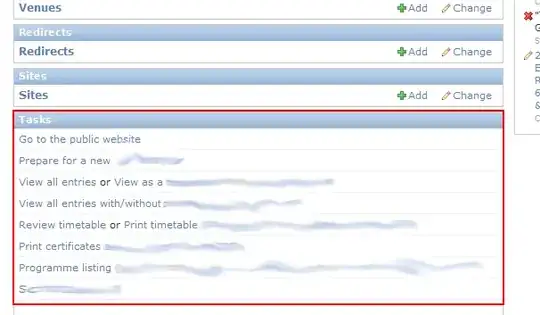
How can I get a clean output per line?