After adding contentMode and scrollsToTop settings along with changes to the insets, I'm no longer seeing the drawing pulling to the top-left:
canvasView.contentMode = .center
canvasView.scrollsToTop = false
The drawing area in Pencil Kit is transparent. No setting exists for background color at this time apparently. Using PKDrawing(data:) appears to be the only way to create a background color as part of the PKDrawing. PKDrawing(data:) is used to initialize the drawing area with a previously created drawing. Thus, a drawing can be created with the code above and saved into a file(Data format) to be used as a background.
The following code is an example of saving a PKDrawing to a Data file by providing a file name:
func saveDrawing(_ fileName: String) {
let data = canvasView.drawing.dataRepresentation()
let fileURL = try! FileManager.default
.url(for: .documentDirectory, in: .userDomainMask, appropriateFor: nil, create: false)
.appendingPathComponent(fileName)
print(fileURL)
do {
try data.write(to: fileURL, options: .atomic)
} catch {
print(error)
}
}
}
Usage:
saveDrawing("whiteBg")
Once the file(drawing) is saved look for the file name in the simulator's document directory. Use the printed fileURL to locate the file. The created file can then be dragged into the project's asset catalog and used as a background image to initialize new drawings.
The following code can be used to import the Data file of the drawing into a new PKDrawing:
func importDrawing(_ fileName: String) -> PKDrawing? {
guard let data = NSDataAsset(name: fileName)?.data else { return nil }
if let drawing = try? PKDrawing(data: data) {
return drawing
} else {
return nil
}
}
Usage:
if let background = importDrawing("whiteBg") {
canvasView.drawing = background
}
Result:
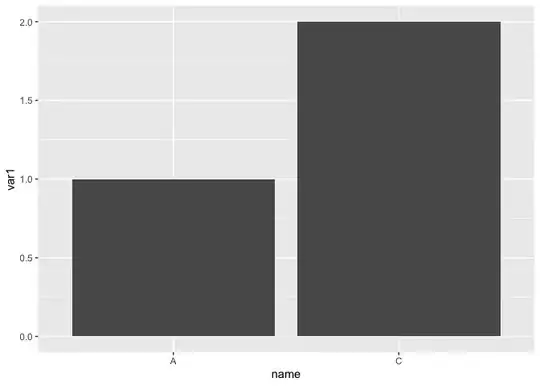
The complete updated code:
import SwiftUI
import PencilKit
struct ContentView: View {
@State var canvasView = PKCanvasView()
var body: some View {
VStack {
Button("SAVE") {
saveDrawing("whiteBg")
}
.frame(height: 50)
CanvasView(canvasView: $canvasView)
}
}
func saveDrawing(_ fileName: String) {
let data = canvasView.drawing.dataRepresentation()
let fileURL = try! FileManager.default
.url(for: .documentDirectory, in: .userDomainMask, appropriateFor: nil, create: false)
.appendingPathComponent(fileName)
print(fileURL)
do {
try data.write(to: fileURL, options: .atomic)
} catch {
print(error)
}
}
}
struct CanvasView {
@Binding var canvasView: PKCanvasView
@State var toolPicker = PKToolPicker()
}
extension CanvasView: UIViewRepresentable {
func makeUIView(context: Context) -> PKCanvasView {
// canvas
canvasView.contentSize = CGSize(width: 1500, height: 1000)
canvasView.drawingPolicy = .anyInput
canvasView.minimumZoomScale = 0.2
canvasView.maximumZoomScale = 4.0
canvasView.backgroundColor = UIColor(red : 0.9, green : 0.9, blue : 0.9, alpha : 1.0)
canvasView.contentInset = UIEdgeInsets(top: 280, left: 400, bottom: 280, right: 400)
canvasView.contentMode = .center
canvasView.scrollsToTop = false
if let whiteBackground = importDrawing("whiteBg") {
canvasView.drawing = whiteBackground
}
canvasView.becomeFirstResponder()
//toolpicker
toolPicker.setVisible(true, forFirstResponder: canvasView)
toolPicker.addObserver(canvasView)
return canvasView
}
func importDrawing(_ fileName: String) -> PKDrawing? {
guard let data = NSDataAsset(name: fileName)?.data else { return nil }
if let drawing = try? PKDrawing(data: data) {
return drawing
} else {
return nil
}
}
func updateUIView(_ uiView: PKCanvasView, context: Context) {}
}