Here is Sheet AA
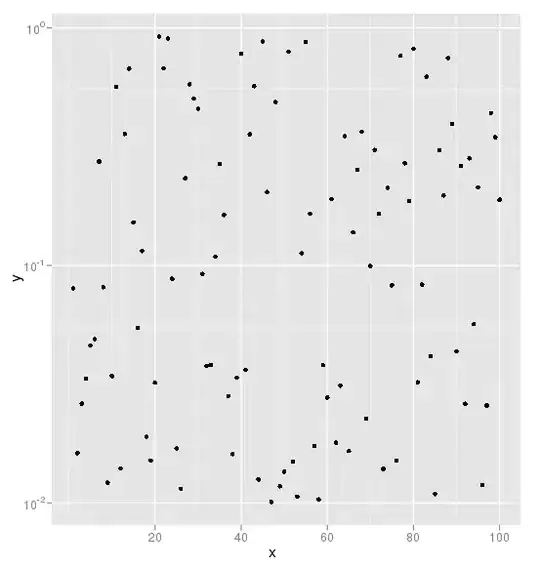
Here is Sheet BB
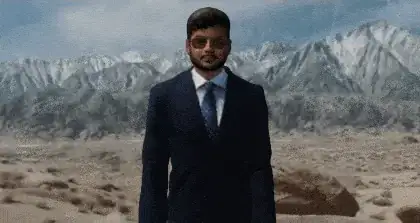
The code:
Option Explicit
Sub Go_Over_Names()
'Store the Workbook
Dim Wrk As Workbook: Set Wrk = ThisWorkbook
'This vars AA and BB to store the sheets
Dim BB As Worksheet: Set BB = Wrk.Worksheets("BB")
Dim AA As Worksheet: Set AA = Wrk.Worksheets("AA")
'Here store the range with the names in each cell in the Sheet BB
Dim NmsRng As Range: Set NmsRng = BB.Range("A1:A4")
'To use it in the for loop
Dim i As Range
Dim Rng As Range
'to store the range name and the sheet where the range exists
Dim NmStr As String
Dim NmSht As String
'To store the sheet where the name exists
Dim Sht As Worksheet
'Let's work... Go the Sheet (BB) where the names (Strings) exist
BB.Activate
'Loop over the cells with the 150 names... Just 3 but you know...
For Each i In NmsRng
'Store the name range into the var
NmStr = i.Value
'Sice the name ranges have the scope for the whole workbook, we can
'take advantage of that.
'if not, it won't be any trouble.
Set Rng = Range(NmStr)
'Here we take the name of the sheet where the actual range exist...
NmSht = Rng.Parent.Name
Set Sht = Wrk.Worksheets(NmSht)
'Let's go to that sheet
Sht.Activate
'Here you can do whatever you want with your range...
'select...
' As @pgSystemTester say, the Select statement, is not good the most of the times
'Using the Rng var, you can copy, change value, loop and anything else
'over the name range.
Rng.Select
'Change the value.
Rng.Value = 1
Next i
End Sub
Result:
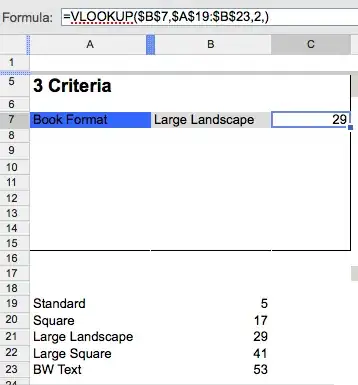
NOTE:
The Name Range EE_004
had scope just to sheet AA
, so, it won't be any trouble if the range is global (Workbook) or just on the Worksheet. The other ranges are global.