'9'
and 9
are both integer constants.
- 9 has integer value of nine
'9'
- has integer value of the (usually) ASCII code of symbol '9'
. It is 57
It is for our convenience when we deal with strings and characters.
char c = '9';
int a = 44;
int b = a - c;
char
is also integer type having size 1 (sizeof(char)
is by definition 1
) Variable c
has integer value of 57.
c
is being converted to int
and it is subtracted from a
(44-57
). The result is assigned to b
ASCII table:
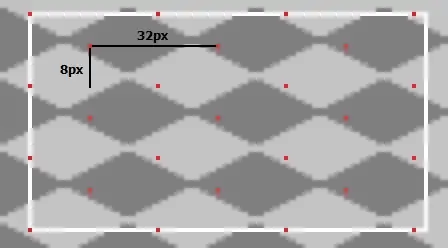
There are other coding conventions and standards (like EBCDIC), but it is very unlikely nowadays to work on the machine which uses them