If what you have as the source array is an actual 3-dimensional array, then what you want is not supported. Indexers on multi-dimensional arrays have multiple parameters. That means, you need to pass them all when trying to access elements on them.
But, you could actually accomplish what you need by manually copying from the 3d array to a 2d one. In order to make this more reusable you could encapsulate this behavior in an extension method that will abstract this away and make your code cleaner and readable.
public static class ArrayExtensions
{
public static T[,] Extract2DArray<T>(this T[,,] array3d, int index = 0)
{
var dim0Length = array3d.GetLength(0);
if (index < 0 || index >= dim0Length)
throw new ArgumentOutOfRangeException(nameof(index), index, $"The index to extract from the 3-d array is out of range. Must be in the range [{0}..{dim0Length - 1}]");
var dim1Length = array3d.GetLength(1);
var dim2Length = array3d.GetLength(2);
T[,] array2d = new T[dim1Length, dim2Length];
for (int i = 0; i < dim1Length; i++)
for (int j = 0; j < dim2Length; j++)
array2d[i, j] = array3d[index, i, j];
return array2d;
}
}
With the above in place, you could use it like this:
string[,,] array3d = new string[,,]
{
{
{ "one", "two", "three" },
{ "four", "five", "six" },
{ "seven", "eight", "nine" },
},
{
{ "ten", "eleven", "twelve" },
{ "thirdteen", "fourteen", "fifteen" },
{ "sixteen", "seventeen", "eighteen" }
},
{
{ "nineteen", "twenty", "twenty one" },
{ "twenty two", "twenty three", "twenty four" },
{ "twenty five", "twenty six", "twenty seven" }
}
};
// Extract the first index:
var first2dArray = array3d.Extract2DArray(0);

// Extract the last 2d array
var last2dArray = array3d.Extract2DArray(2);
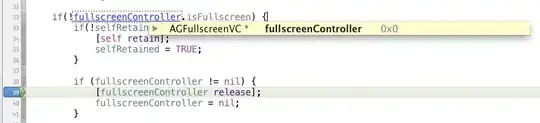
// Trying to use this with invalid indexes would throw an exception
var invalidIndexArray = array3d.Extract2DArray(3); // a negative index will fail too
Exception: The index to extract from the 3-d array is out of range. Must be in the range [0..2] (Parameter 'index')
Actual value was 3.