You've left out a lot of information about what your code is doing, so tough to say what you might be doing wrong.
Here's a quick example of loading a view from a child view controller, rounding its corners and giving it a shadow...
First, an "add child" extension:
extension UIViewController {
func add(_ child: UIViewController) {
addChild(child)
view.addSubview(child.view)
child.didMove(toParent: self)
}
}
next, a simple "StartVC" view controller, with a centered label (for the customer string), along with a Pan gesture to allow it to be dragged:
class StartVC: UIViewController {
var customer: String = ""
private var prevLocation: CGPoint = .zero
init(customer: String) {
self.customer = customer
super.init(nibName: nil, bundle: nil)
}
required init?(coder: NSCoder) {
super.init(coder: coder)
}
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .yellow
let label = UILabel()
label.font = .systemFont(ofSize: 24.0, weight: .light)
label.textAlignment = .center
label.numberOfLines = 0
label.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(label)
let g = view.safeAreaLayoutGuide
NSLayoutConstraint.activate([
label.centerYAnchor.constraint(equalTo: g.centerYAnchor),
label.leadingAnchor.constraint(equalTo: g.leadingAnchor, constant: 20.0),
label.trailingAnchor.constraint(equalTo: g.trailingAnchor, constant: -20.0),
])
label.text = customer
let pg = UIPanGestureRecognizer(target: self, action: #selector(handlePan(_:)))
self.view.isUserInteractionEnabled = true
self.view.addGestureRecognizer(pg)
}
@objc private func handlePan(_ sender: UIPanGestureRecognizer) {
let translation = sender.translation(in: self.view.superview)
self.view.center = CGPoint(x: prevLocation.x + translation.x, y: prevLocation.y + translation.y)
}
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
prevLocation = self.view.center
}
}
and, an example view controller... we add a large-font label that will be underneath the "StartVC view" -- then it loads the child VC, gets its view, rounds the corners and sets shadow properties, and adds it centered on the view where it can then be dragged around:
class DragTestVC: UIViewController {
var slideView: UIView!
override func viewDidLoad() {
super.viewDidLoad()
// let's add a label with a bunch of text
// so we can see the "slide view" moving over it
let label = UILabel()
label.font = .systemFont(ofSize: 60.0, weight: .light)
label.textAlignment = .center
label.numberOfLines = 0
label.textColor = .systemRed
label.backgroundColor = UIColor(white: 0.95, alpha: 1.0)
label.text = "This is just some text for the label so we can see that the \"slide view\" is being moved over other UI elements."
label.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(label)
let g = view.safeAreaLayoutGuide
NSLayoutConstraint.activate([
label.widthAnchor.constraint(equalTo: g.widthAnchor, multiplier: 0.9),
label.centerYAnchor.constraint(equalTo: g.centerYAnchor),
label.centerXAnchor.constraint(equalTo: g.centerXAnchor),
])
let slideVC = StartVC(customer: "Customer\n\nRobert Smith")
add(slideVC)
slideView = slideVC.view
slideView.layer.cornerRadius = 12
slideView.layer.shadowColor = UIColor.black.cgColor
slideView.layer.shadowOffset = CGSize(width: 3, height: 3)
slideView.layer.shadowOpacity = 0.7
slideView.layer.shadowRadius = 4.0
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
// let's set the "slideView" frame to
// 60% of view width
// height 1.5 * its own width
// centered in the view
let width = view.frame.width * 0.6
let height = width * 1.5
slideView.frame = .init(x: 0.0, y: 0.0, width: width, height: height)
slideView.center = view.center
}
}
Looks like this:
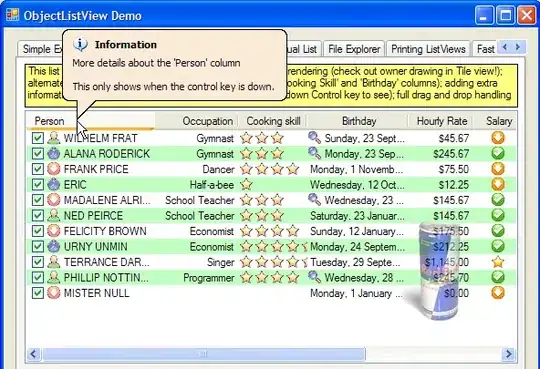