Ok, a good idea, but you have a BOATLOAD of issues here.
First up by creating a ddl in code, then when you "assign" that to the existing control on the web page, you are BLOWING OUT a HUGE amount of settings and setup. Remember, when you drop in that control:
The "designer" file adds that ddl to the page class.
upon running, then a BOATLOAD of settings occur including that of view state, and a WHOLE bunch of connections setup to the current page class. So, all kinds of things will occur. Including you "blowing" out the events that you no doubt would want to have for the ddl (such as SelectedIndexChanged). So, what happens when you add/change events, and other settings to that ddl? Your code going to blow out all those settings - including that of having "view state" attached to the control to operate correctly on that page. So "too many" properties you would have to setup for this to work.
There are two solutions I suggest:
Create a user control, and then simple have some properties and methods - includes stored procedure name. That way, you can just drag + drop from the project explore the control, and you are done.
However, somewhat FAR more simple?
Just pass the current instance of the ddl object to your helper routine.
so, say I have this markup:
<asp:DropDownList ID="DropDownList1" runat="server">
</asp:DropDownList>
Then this code:
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
DropHelper(DropDownList1,
"SELECT id, HotelName FROM tblHotelsA",
"id","hotelname",
"Select a Hotel");
}
}
void DropHelper(DropDownList dl, string strSQL,
string dValue, string dField,
string sFirstLine)
{
dl.DataValueField = dValue;
dl.DataTextField = dField;
using (SqlConnection con = new SqlConnection(Properties.Settings.Default.TEST4))
{
using (SqlCommand cmd = new SqlCommand(strSQL, con))
{
DataTable dt = new DataTable();
con.Open();
dt.Load(cmd.ExecuteReader());
dl.DataSource= dt;
dl.DataBind();
dl.Items.Insert(0, new ListItem(sFirstLine, ""));
}
}
}
So, "pass" the existing control in question. That way its attachment to the current page class object, the viewstate (and a boatload of other settings you would have to code out) are not required to be setup by you. So, creating a whole new instance of that control means you lose all of the code behind settings that visual studio create(s) and sets up for you. (including things like viewstate etc.).
And now we see/get this:
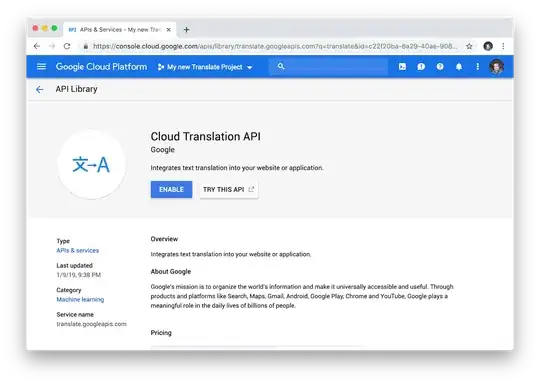