Here is one way to do that in Python/OpenCV.
- Read the input image
- Choose one of your colors and set up lower and upper ranges for them. Then use cv2.inRange() to threshold on that color.
- Then get external contours and draw them as white filled on a black background (usual polarity for masks).
- Optionally invert the mask so you have black regions on a white background
- Save the mask
Input:
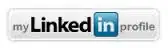
import cv2
import numpy as np
# read the input image
img = cv2.imread('fish_outlines.png')
# specify outline color and threshold on it
# yellow
upper = (50,255,255)
lower = (0,180,180)
thresh = cv2.inRange(img, lower, upper)
# get external contours
contours = cv2.findContours(thresh , cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours = contours[0] if len(contours) == 2 else contours[1]
# draw white filled contours on black background
mask = np.zeros_like(img)
for cntr in contours:
cv2.drawContours(mask, [cntr], 0, (255,255,255), -1)
# invert so black regions on white background if desired
mask = 255 - mask
# save result
cv2.imwrite('fish_outlines_yellow_mask.png', mask)
# show results
cv2.imshow('thresh', thresh)
cv2.imshow('mask', mask)
cv2.waitKey(0)
Resulting Mask:

If you have lists of outline coordinates, that could be used. It would be easy for single regions. For the ones like the yellow with multiple regions, you would need separate lists for each individual yellow region.