I have just written a tool that does that. It's just a few lines of code, and it is not finished yet, but very simple to extend (I might do that tomorrow somewhere).
The result : just click extract from a menu, and the result will be on the clipboard.
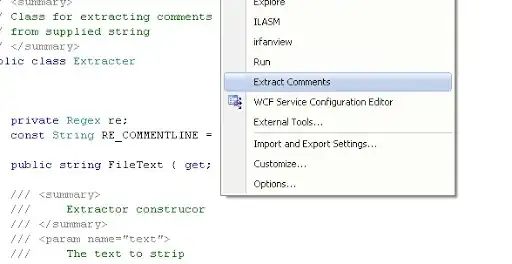
Two very easy steps :
- Write a program that takes a file as argument, takes some text from that file and paste them to the clipboard.
- Integrate that program into your IDE.
STEP 1, part 1
Main program, take a file, send it to an 'extractor' and write results to clipboard.
class Program
{
[STAThread]
static void Main(string[] args)
{
if (args.Length == 0) return;
FileInfo f = new FileInfo(args[0]);
Extracter e = new Extracter(f.OpenText().ReadToEnd());
Clipboard.SetText(e.GetExtractedText());
}
}
STEP 1, part 2
The extracter : get all the textpatterns you need out of it using regex of course, and return the conforming string. I have ommitted comments for density of post, and since the principle is simple and explained already.
public class Extracter
{
private Regex re;
// extend/adapt regex patterns for better result.
const String RE_COMMENT_AND_NEXT_LINE= @"(?<=([\/]{3})).+";
public string FileText { get; set; }
public Extracter(String FileText)
{
this.FileText = FileText;
}
public String GetExtractedText()
{
StringBuilder sb = new StringBuilder(String.Empty);
re = new Regex(RE_COMMENT_AND_NEXT_LINE);
foreach (Match match in re.Matches(FileText))
{
sb.Append(match.ToString());
}
return sb.ToString();
}
}
STEP 2 : Add to IDE
That IDE dependent of course, but always easy. See my screenshot for VS2008 :
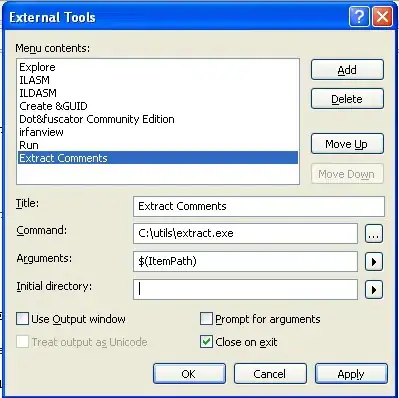