If a shared access signature is generated by an authorized user, a valid link with the token is appended to the blob's tail, then everyone can view it with no trouble (including unauthorized users). This is unexpected.
You can restrict the blob container by generating an SAS token with IP address.
To ensure that only the authorized user can access the blob with the generated SAS token, you need to set the appropriate permissions on the SAS token. Specifically, you should set the Read
permission on the token and also set the IPRange
property to the IP address of the authorized user.
Initially, I set up an IP address for the storage account.
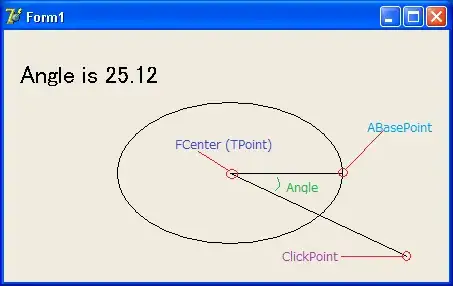
You can use the below code to generate a SAS token with Authorized IP address.
Code:
using Azure.Storage.Blobs;
using Azure.Storage.Sas;
using System;
using System.Net;
namespace BlobSasDemo
{
class Program
{
static void Main(string[] args)
{
// Replace these values with your own connection string, container name, blob name, and authorized user's IP address
string connectionString = "Your connection string";
string containerName = "test2";
string blobName = "test.png";
string authorizedIpAddress = "103.xx.xxx.xx";
// Create a BlobClient instance
BlobClient blobClient = new BlobClient(connectionString, containerName, blobName);
// Create a BlobSasBuilder instance
BlobSasBuilder sasBuilder = new BlobSasBuilder()
{
BlobContainerName = containerName,
BlobName = blobName,
Resource = "b",
StartsOn = DateTime.UtcNow.AddMinutes(-5),
ExpiresOn = DateTime.UtcNow.AddMinutes(10),
IPRange = new SasIPRange(IPAddress.Parse(authorizedIpAddress)),
};
// Set the permissions to only allow read access
sasBuilder.SetPermissions(BlobSasPermissions.Read);
// Generate the SAS token
Uri sasTokenUri = blobClient.GenerateSasUri(sasBuilder);
// Print the SAS token URI
Console.WriteLine("SAS token URI: {0}", sasTokenUri);
Console.ReadLine();
}
}
}
Output:
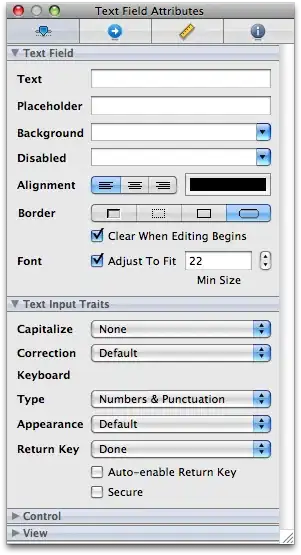
If we try with a different Ip address you will get an error like below:
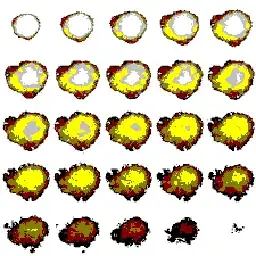
Reference:
AccountSasBuilder Class (Azure.Storage.Sas) - Azure for .NET Developers | Microsoft Learn