The easiest is probably to create a DTO that matches the payload you expect and then set the type to ulong
of the seed property.
Here is an example DTO:
public class Seeder {
public ulong Seed {get;set;}
}
And here is how you use it, instead of Parse:
void Main()
{
var str = @"{""seed"":11405418255883340000}";
var ser = new JsonSerializer();
var jr = new JsonTextReader(new StringReader(str));
var seed = ser.Deserialize<Seeder>(jr);
seed.Dump();
}
This is what LinqPad shows me:
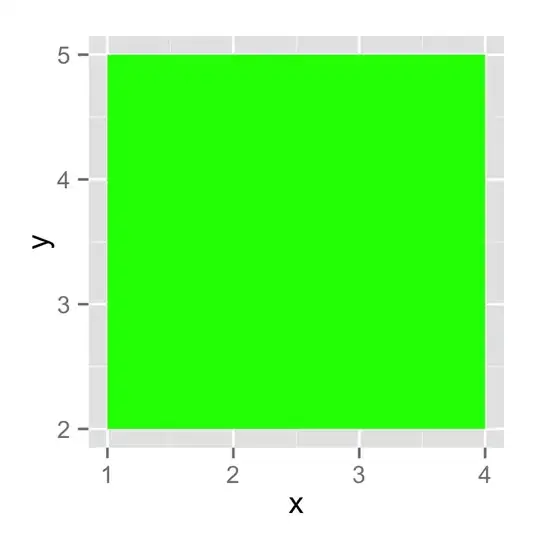
If I change the property type back to long
I get this exception:
Error converting value 11405418255883340000 to type 'System.Int64'. Path 'seed', line 1, position 28.
Note that you don't have to code a fully specified DTO object. This one works as wel, where we simply don't have the seed property on our DTO class:
void Main()
{
var str = @"{""seed"":11405418255883340000, ""bar"": 4}";
var ser = new JsonSerializer();
var jr = new JsonTextReader(new StringReader(str));
var seed = ser.Deserialize<Seeder>(jr);
seed.Dump();
}
public class Seeder {
public int Bar {get;set;}
}
If you are lazy you can drop the JSON payload into an online class generator and/or tooling in your IDE. See for a starting point on that: https://stackoverflow.com/a/21611680