tl;dr
Use date.getTime()
for comparisons.
Based on my testing, it was at least 25% than the next fastest alternative (date.valueOf()
).
Details
Came across this in 2022. As others have already said, comparing like date1.getTime() === date2.getTime()
is the way to go.
Someone else shared a jsperf link in an answer that seems broken for me right now, so decided to add some performance comparison of my own.
I created two arrays containing 1000 dates each. All dates will naturally be different instances (meaning direct ===
checks will fail), so what this benchmark does, is test what the fastest way is to convert a date to a primitive.
Here's the test data:
const data1 = Array.from({length: 1000}, () => new Date())
const data2 = Array.from({length: 1000}, () => new Date())
And here are the test cases (there's more in the link below):
// 1
data1.forEach((d1, i) => d1.getTime() === data2[i].getTime());
// 2
data1.forEach((d1, i) => d1.valueOf() === data2[i].valueOf());
// 3
data1.forEach((d1, i) => Number(d1) === Number(data2[i]));
// 4
data1.forEach((d1, i) => d1.toISOString() === data2[i].toISOString());
Result (use date.getTime()
)
Not a surprise that a date.getTime()
conversion is much faster. It's around 25% faster than date.valueOf()
, and between 10x and 100x faster than everything else (as far as I've checked).
Additionally, introducing optional chaining slowed the best case by almost 10% for me. Found that interesting. date.valueOf()
also slowed down by 5% compared to its non optional chaining counterpart.
data1.forEach((d1, i) => d1?.getTime() === data2[i]?.getTime());
Benchmark link: here
Here's an image, in case the above link breaks at some point in the future.
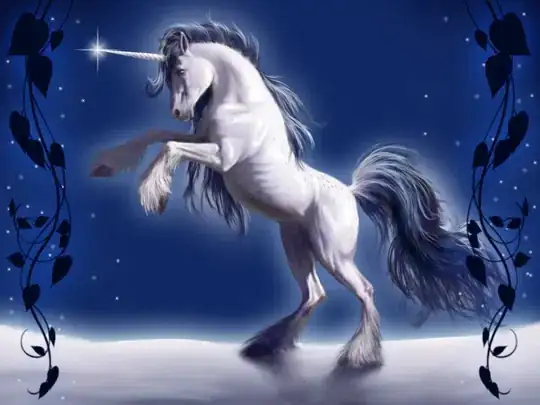