So you have a set of parameters and two states of them (either they enabled or not). That means that you have 2^n
combinations where n
is the total amount of your parameters.
Hence the solution would be to iterate from 0
to 2^n-1
, convert the current number into binary representation and build your RequestSpecification
accordingly.
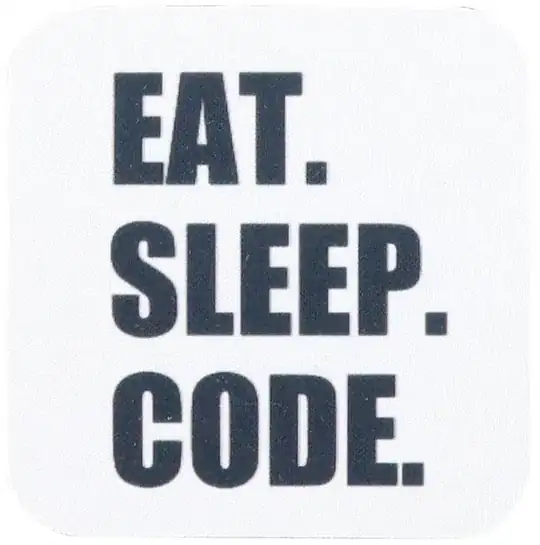
Let's break by steps.
0. Lets have the Parameter
class that would hold our key-value
class Parameter {
String key;
Object value;
public Parameter(String key, Object value){
this.key = key;
this.value = value;
}
public String getKey() {
return key;
}
public Object getValue() {
return value;
}
}
1. Convert a number to binary representation. See this advice.
boolean[] getCombination(int rowNum, int bits){
boolean[] flags = new boolean[bits];
for (int i = bits - 1; i >= 0; i--) {
flags[i] = (rowNum & (1 << i)) != 0;
}
return flags;
}
2. Now we can build a flag mask that would hold enable/disable flag for all combinations
boolean[][] getCombinations(Parameter[] parameters){
int rowNum = (int)Math.pow(2, parameters.length);
boolean[][] results = new boolean[rowNum][parameters.length];
for(int i = 0; i < rowNum; i++){
boolean[] line = getCombination(i, parameters.length);
for(int j = 0; j < parameters.length; j++){
results[i][j] = line[j];
}
}
return results;
}
3. Finally we can generate RequestSpecification
with required mask
RequestSpecification generateCombination(RequestSpecification original,
Parameter[] parameters,
boolean[] flags){
RequestSpecification changed = original;
for(int i = 0; i < parameters.length; i++){
Parameter p = parameters[i];
if(flags[i]){
changed = changed.queryParam(p.getKey(), p.getValue());
}
}
return changed;
}
-- How to use all that
Assume that you have all these methods implemented in ParamTest
class. Then you could test the solution by adding following main method to it:
public static void main(String[] args) {
ParamTest paramTest = new ParamTest();
Parameter[] parameters = {
new Parameter("name1", "val1"),
new Parameter("name2", 123),
new Parameter("name3", List.of("a", "b", "c"))
};
RequestSpecification originalSpec = RestAssured.given();
boolean[][] mask = new ParamTest().getCombinations(parameters);
for(int i = 0; i < mask.length; i++){
if(mask[i].length != parameters.length){
throw new IllegalStateException("Unexpected size of array");
}
paramTest
.generateCombination(originalSpec, parameters, mask[i])
.get() // Do here what you want;
;
}
}
So that:
- You create an instance of the class
- You define the list of parameters
- You prepare original
RequestSpecification
that you'll be customizing in further loop
- You obtain flag mask array
- You iterate through that flag mask array, obtain particular customized specification that has only certain set of parameters and apply the check that you want.