When the button is disabled, it will not generate mouse events.
There is a simple trick to accomplish what you want:
Place the button in a container-control which is marginally larger than the button (e.g. a panel) and attach the tooltip to this control.
When enabling the button, you disable the tooltip. When disabling the button you activate the tooltip.
A small example using winforms :
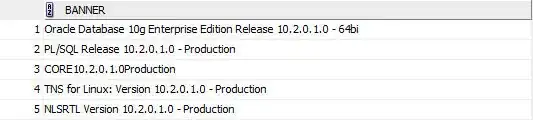
where the button is placed inside panel1
and a 'tooltip1' is attached to that panel with text 'Button Disabled'.
In the event handler for the checkbox you change the state of both the button and the tooltip:
private void checkBox1_CheckedChanged(object sender, EventArgs e)
{
if (checkBox1.Checked)
{
button1.Enabled = true;
toolTip1.Active = false;
return;
}
button1.Enabled = false;
toolTip1.Active = true;
}
The same mechanism will work in WPF as well. Here an invisible border is used as container:
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="294" Width="413">
<StackPanel Orientation="Vertical" HorizontalAlignment="Center" VerticalAlignment="Center">
<CheckBox Content="Button Enabled" Click="CheckBox_Changed" Margin="20" />
<Border x:Name ="border1" BorderThickness="0" Height="40" Padding="10" Width="220" >
<Border.ToolTip>
<TextBlock>Button.Disabled</TextBlock>
</Border.ToolTip>
<Button x:Name="button1" Content="button1" HorizontalAlignment="Center" Width ="200" IsEnabled= "False" />
</Border>
</StackPanel>
With the code-behind:
public partial class MainWindow : Window
{
TextBlock buttonTooltip = new TextBlock { Text = "Button Disabled."};
public MainWindow()
{
InitializeComponent();
}
private void CheckBox_Changed(object sender, RoutedEventArgs e)
{
var checkBox = sender as CheckBox;
var tooltip = border1.ToolTip as TextBlock;
if (checkBox.IsChecked ?? false)
{
button1.IsEnabled = true;
border1.ToolTip = null;
return;
}
button1.IsEnabled = false;
border1.ToolTip = buttonTooltip;
}
}
Update: The OP's question is better solved in the link provided by ASh below. Still the technique shown here can be usefull in other situations where you need to process input events on a control that does not provide them itself.