TLDR; Your PNG has been exported using a Palette (e.g. 256 colors) to reduce the overall file size. It is possible that the transparency information may not be preserved accurately. This is because the palette-based color reduction technique is primarily designed for preserving color information and not transparency. To ensure that transparency is maintained, it is generally recommended to export PNG images with full color support (32-bit RGBA) instead of using a palette with limited colors.

You may try the RGBA format and the code that you find further down below.
Disclaimer: I'm using PHP 8.1.6 along with libPNG: 1.6.37 on macOS (Intel, Ventura 13.2.1 (22D68)) and it works just fine.
Preconditions:
I've ensured that your frames are 100% transparent first in Affinity Photo I've manually deleted these areas with a flood-fill tool. I've also exported the image with the default settings. If I use your image, I'll end up with an alpha value of 229.

Display in browser / mime-type:
The browser adds some styles and classes to the image when rendering with header('Content-Type: image/png')
(default styles in the browser). We have only requested the browser to render the isolated image, but the browser adds some more stuff to make it look "good" - see the DOM in the screenshots.
In Firefox for example you can see this "transparent" class that is added - it adds a background (I have disabled this in the first screenshot). If we change it to red you can see that the background now is red so the frames are red too because they are 100% transparent.
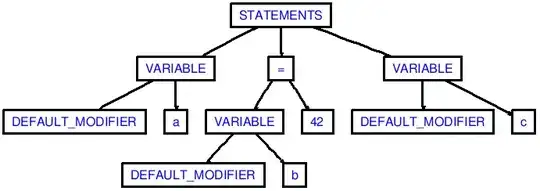
So how do we fix this? Depends on what you are trying to do. Basically, you can just render your own HTML-Document instead of using the mime-type: image/png
so there is no browser default.
E.g.
<?php
// ini_set("display_errors", 1);
// error_reporting(-1);
$image = imagecreatefrompng('./test-img.png');
imagealphablending($image, true); // Make sure the alpha channel is supported
$trimmed = imagecropauto($image, IMG_CROP_SIDES);
$generatedImagePath = './test-img-generated.png';
if ($trimmed !== false) {
imagesavealpha($trimmed, true); // Make sure the alpha channel gets exported when you save it
imagepng($trimmed, $generatedImagePath);
} else {
echo "Failed to crop the image.";
}
imagedestroy($image); // Frees memory prior to PHP 8.0
imagedestroy($trimmed); // ...
?>
<!DOCTYPE html>
<html>
<body style="background: transparent; background-image: url('./pattern.jpg')">
<img src="<?php echo $generatedImagePath; ?>" style="width: 480px; aspect-ratio: 1;">
</body>
</html>
Renders:
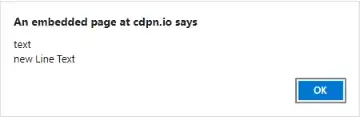