How to implement cooldowns for discord.py
We can use the decorator: @discord.app_commands.checks.cooldown(rate, per, *, key=...)
on our slash command to add a cooldown.
The rate
is the:
The number of times a command can be used before triggering a cooldown.
The per
is the:
The amount of seconds to wait for a cooldown when it’s been triggered.
Example
import discord
from discord import app_commands # to use in app commands
intents = discord.Intents.default()
intents.message_content = True
client = discord.Client(command_prefix="!", intents=intents) # Create client
tree = app_commands.CommandTree(client) # Set up application tree
@discord.app_commands.checks.cooldown(1, 3) # add cooldown for every command three seconds of cooldown
@tree.command(name="cooldowntest", description="Test the cooldown", guild=discord.Object(
id=yourguildid))
async def first_command(interaction):
await interaction.response.send_message("Worked!")
@first_command.error # Tell the user when they've got a cooldown
async def on_test_error(interaction: discord.Interaction, error: app_commands.AppCommandError):
if isinstance(error, app_commands.CommandOnCooldown):
await interaction.response.send_message(str(error),
ephemeral=True) # https://discordpy.readthedocs.io/en/stable/interactions/api.html?highlight=checks%20cooldown#discord.app_commands.checks.cooldown
@client.event
async def on_ready():
await tree.sync(guild=discord.Object(guildidhere)) # sync tree
print(f'We have logged in as {client.user}')
Output
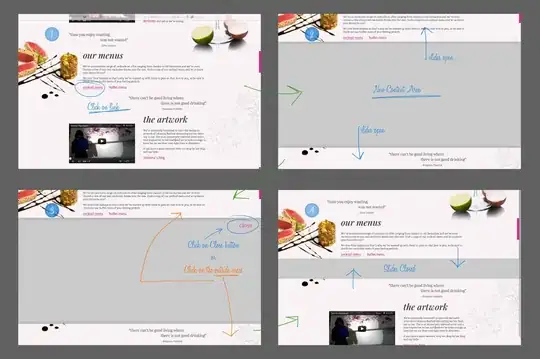
Resources