There is a more modern and elegant way to implement it unless you
prefer not to use Material library. But if you do prefer, you will
benefit from its convenience. So you choose...
Here is two photos showing the end result both collapsed and expanded state.
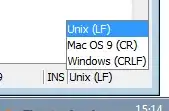
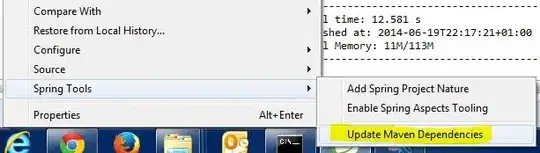
Well, if you'd like to implement in this way, you should be migrated your
project to AndroidX via the Refactor > Migrate to AndroidX menu option
in Android Studio and also have added the material library along with cardview
library in order to be able to build your project with the implementation that
I'll show next. So in your module's build.gradle file you shoud have the
following dependencies:
dependencies {
...
implementation 'com.google.android.material:material:1.9.0'
implementation 'androidx.cardview:cardview:1.0.0'
...
}
Next you wanna shape your bottom sheet with round corners but only the
top corners. To do this you need to add a ShapeAppearanceOverlay
style in
your styles resource file as following:
styles.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
...
<style name="ShapeAppearanceOverlay.RoundCorners.Top"
parent="ShapeAppearance.MaterialComponents.LargeComponent">
<item name="cornerFamily">rounded</item>
<item name="cornerSizeTopLeft">20dp</item>
<item name="cornerSizeTopRight">20dp</item>
<item name="cornerSizeBottomLeft">0dp</item>
<item name="cornerSizeBottomRight">0dp</item>
</style>
...
</resources>
You can adjust each corner's value with this style and also you can use a cut
cornerFamily
if you wish.
Next step is to implement the bottom sheet's layout. You can implement it
either independent to make it reusable or embedded to use it directly in your
main layout. I implemented as independent here. I used a MaterialCardView
as
root element in order to be able to apply the ShapeAppearance
and as well as
to control elevation more conveniently. Because the elevation is the key property
here to control the element's shadow.
bottom_sheet.xml
<?xml version="1.0" encoding="utf-8"?>
<com.google.android.material.card.MaterialCardView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:cardElevation="16dp"
app:cardMaxElevation="16dp"
app:layout_behavior="@string/bottom_sheet_behavior"
app:shapeAppearanceOverlay="@style/ShapeAppearanceOverlay.RoundCorners.Top"
app:behavior_peekHeight="56dp">
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="32dp"
android:layout_marginBottom="16dp"
android:text="OK"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="32dp"
android:text="Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum."
android:textAlignment="center"
app:layout_constraintBottom_toTopOf="@+id/button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="32dp"
android:text="Bottom Sheet Example"
android:textAlignment="center"
android:textAppearance="@style/TextAppearance.AppCompat.Body1"
android:textSize="16sp"
app:layout_constraintBottom_toTopOf="@+id/textView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
</com.google.android.material.card.MaterialCardView>
And finally you include the bottom sheet to your container layout. However note
that the bottom sheet's parent layout must be a CoordinatorLayout
so that
it can behave with its bottom sheet behaviors.
container.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.coordinatorlayout.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior" />
<include
android:id="@+id/bottomSheet"
layout="@layout/bottom_sheet"/>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
After implementing everything properly you should see the same result as in the first 2 photos.